根据给定单词的出现频度,构造一棵哈夫曼树,并输出对应的哈夫曼编码(C语言实现)。 单词 A B C D E F G H 出现频度 9 5 3 7 6 2 1 1
时间: 2023-06-04 09:02:46 浏览: 91
好的,关于你的问题,我可以提供以下代码示例以构造哈夫曼树并输出哈夫曼编码(使用C语言):
```
#include<stdio.h>
#include<malloc.h>
#define MAXBIT 100
#define MAXVALUE 10000
#define MAXLEAF 30
#define MAXNODE MAXLEAF*2 -1
typedef struct
{
float weight;
int parent;
int lchild;
int rchild;
char code[MAXBIT];
} HNodeType;
void HuffmanTree(HNodeType HuffNode[MAXNODE], int n)
{
int i,j,k;
float min1,min2;
int x1,x2;
for (i=0;i<2*n-1;i++)
{
HuffNode[i].weight=0;
HuffNode[i].parent=-1;
HuffNode[i].lchild=-1;
HuffNode[i].rchild=-1;
}
for (i=0;i<n;i++)
{
printf("请输入字母:");
fflush(stdin);
scanf("%c",&HuffNode[i]. code[0]);
printf("请输入权重:");
fflush(stdin);
scanf("%f",&HuffNode[i]. weight);
}
for (i=0;i<n-1;i++)
{
min1=min2=MAXVALUE;
x1=x2=0;
for (j=0;j<n+i;j++)
{
if (HuffNode[j].weight<min1 && HuffNode[j].parent==-1)
{
min2=min1;
x2=x1;
min1=HuffNode[j].weight;
x1=j;
}
else if (HuffNode[j].weight<min2 && HuffNode[j].parent==-1)
{
min2=HuffNode[j].weight;
x2=j;
}
}
HuffNode[x1].parent=n+i;
HuffNode[x2].parent=n+i;
HuffNode[n+i].weight=HuffNode[x1].weight+HuffNode[x2].weight;
HuffNode[n+i].lchild=x1;
HuffNode[n+i].rchild=x2;
}
}
void HuffmanCode(HNodeType HuffNode[MAXNODE], int n)
{
int i,j,c,p;
char cd[MAXBIT];
int start;
cd[n-1]='\0';
for (i=0;i<n;i++)
{
start=n-1;
for (c=i,p=HuffNode[i].parent;p!=-1;c=p,p=HuffNode[p].parent)
{
if (HuffNode[p].lchild==c)
cd[--start]='0';
else
cd[--start]='1';
}
strcpy(HuffNode[i].code,&cd[start]);
}
}
int main()
{
HNodeType HuffNode[MAXNODE];
int n;
printf("请输入叶节点个数n:");
scanf("%d",&n);
HuffmanTree(HuffNode,n);
HuffmanCode(HuffNode,n);
printf("\n\n\n哈夫曼树如下所示:\n");
for (int i=0; i<2*n-1; i++)
{
printf("%d %c %f %d %d %d\n",i+1,HuffNode[i]. code[0],HuffNode[i].weight,
HuffNode[i]. parent,HuffNode[i].lchild,HuffNode[i].rchild);
}
printf("\n\n\n哈夫曼编码如下所示:\n");
for (int i=0; i<n; i++)
{
printf("%c的哈夫曼编码为:%s\n",HuffNode[i]. code[0],HuffNode[i].code);
}
return 0;
}
```
希望这可以回答你的问题。如果你有任何其他问题,可以随时问我!
相关推荐
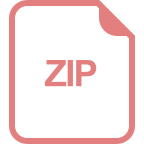














