Problem B. 小朋友的 mex 题目英文名:mex 时空限制:1s/256MB 题目描述: 函数 mex(a,b,c......)表示在 a,b,c......中最小的没有出现的自然数 。 有 n 个小朋友,每个小朋友有一个数,分别为a[1] 到 a[n] 。 小朋友们想求出: 对于 a 数组的所有连续子区间,求出它们的 mex的和。 由于答案的极限大小大约是 10e15 ,小朋友们就不要求你取模了。 (居然提醒大家开 long long ,多么良心的出题人!) C++代码实现
时间: 2024-04-10 13:34:53 浏览: 158
Sure! Here's a C++ implementation for the problem:
```cpp
#include <iostream>
#include <vector>
using namespace std;
long long mex(vector<int>& a) {
int n = a.size();
vector<bool> visited(n+1, false);
for (int num : a) {
if (num >= 0 && num <= n) {
visited[num] = true;
}
}
for (int i = 0; i <= n; i++) {
if (!visited[i]) {
return i;
}
}
return n + 1;
}
long long sumMex(vector<int>& a) {
int n = a.size();
long long sum = 0;
for (int i = 0; i < n; i++) {
for (int j = i; j < n; j++) {
vector<int> subArray(a.begin() + i, a.begin() + j + 1);
sum += mex(subArray);
}
}
return sum;
}
int main() {
int n;
cin >> n;
vector<int> a(n);
for (int i = 0; i < n; i++) {
cin >> a[i];
}
long long result = sumMex(a);
cout << result << endl;
return 0;
}
```
This code defines two functions: `mex` and `sumMex`. The `mex` function finds the minimum non-negative integer that is not present in the given array. The `sumMex` function calculates the sum of mex values for all the contiguous subarrays of the input array. The main function reads the input, calls `sumMex`, and prints the result.
Please note that this implementation assumes that the input array contains non-negative integers. If the array can contain negative integers as well, you may need to modify the code accordingly.
Let me know if you have any further questions!
阅读全文
相关推荐
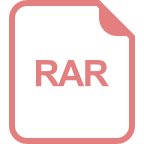
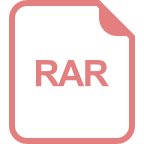
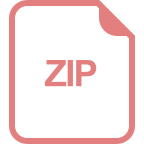
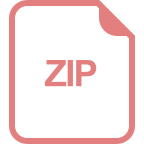
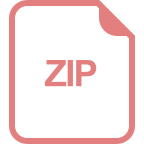
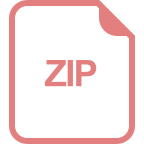
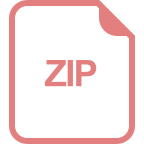
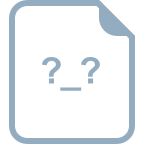
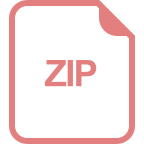
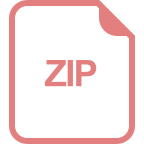
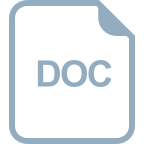
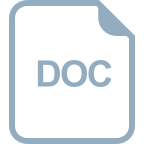
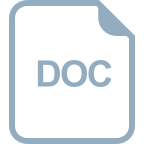
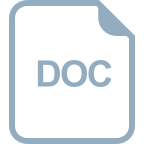




