vue通过实现大文件切片上传
时间: 2024-01-01 14:04:51 浏览: 29
Vue可以通过以下步骤实现大文件切片上传:
1. 将大文件切成多个小文件。可以使用JavaScript中的Blob.slice()方法将文件分成多个块。
2. 使用XMLHttpRequest对象将每个块上传到服务器。可以使用Vue的axios库来发送HTTP请求。
3. 在服务器端将每个块合并成一个完整的文件。可以使用Node.js的fs模块来处理文件操作。
4. 如果需要,可以在上传过程中显示上传进度。可以使用Vue的组件来显示进度条。
以下是一个示例代码:
```
<template>
<div>
<input type="file" @change="handleFileChange" />
<button @click="uploadFile">Upload</button>
<div v-if="uploading">
Uploading... {{ progress }}%
</div>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
file: null,
uploading: false,
progress: 0,
};
},
methods: {
handleFileChange(event) {
this.file = event.target.files[0];
},
async uploadFile() {
const CHUNK_SIZE = 1000000; // 1MB
const fileSize = this.file.size;
const chunks = Math.ceil(fileSize / CHUNK_SIZE);
let currentChunk = 0;
this.uploading = true;
while (currentChunk < chunks) {
const start = currentChunk * CHUNK_SIZE;
const end = Math.min(start + CHUNK_SIZE, fileSize);
const chunk = this.file.slice(start, end);
const formData = new FormData();
formData.append('file', chunk);
formData.append('chunk', currentChunk);
formData.append('chunks', chunks);
await axios.post('/upload', formData, {
onUploadProgress: (progressEvent) => {
this.progress = Math.round((100 * progressEvent.loaded) / progressEvent.total);
},
});
currentChunk++;
}
this.uploading = false;
this.progress = 0;
},
},
};
</script>
```
在服务器端,可以使用以下代码来处理文件上传和合并:
```
const fs = require('fs');
const path = require('path');
const express = require('express');
const app = express();
app.post('/upload', (req, res) => {
const file = req.files.file;
const chunk = parseInt(req.body.chunk);
const chunks = parseInt(req.body.chunks);
const fileName = file.name;
const filePath = path.join(__dirname, 'uploads', fileName);
const stream = fs.createWriteStream(filePath, { flags: chunk === 0 ? 'w' : 'a' });
stream.on('finish', () => {
if (chunk === chunks - 1) {
// All chunks have been uploaded. Merge the file.
const mergedFilePath = path.join(__dirname, 'uploads', `merged-${fileName}`);
const mergedStream = fs.createWriteStream(mergedFilePath);
for (let i = 0; i < chunks; i++) {
const chunkFilePath = path.join(__dirname, 'uploads', `${fileName}.${i}`);
const chunkStream = fs.createReadStream(chunkFilePath);
chunkStream.pipe(mergedStream, { end: false });
chunkStream.on('end', () => {
fs.unlink(chunkFilePath, (error) => {
if (error) {
console.error(`Error deleting chunk file ${chunkFilePath}: ${error.message}`);
}
});
});
}
mergedStream.on('finish', () => {
res.status(200).send('File uploaded successfully!');
});
mergedStream.on('error', (error) => {
console.error(`Error merging file: ${error.message}`);
res.status(500).send('Error merging file');
});
} else {
res.status(200).send('Chunk uploaded successfully!');
}
});
stream.on('error', (error) => {
console.error(`Error writing chunk file: ${error.message}`);
res.status(500).send('Error writing chunk file');
});
file.pipe(stream);
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
相关推荐
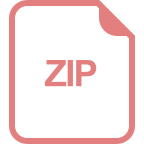














