python用turtle写自己名字
时间: 2023-05-31 15:18:23 浏览: 356
### 回答1:
以下是用Python的turtle模块写自己名字的示例代码:
```python
import turtle
# 创建一个画布和画笔
canvas = turtle.Screen()
pen = turtle.Turtle()
# 设置画笔的颜色和粗细
pen.color("blue")
pen.pensize(5)
# 写出自己的名字
pen.penup()
pen.goto(-100, )
pen.pendown()
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.right(90)
pen.forward(50)
pen.left(90)
pen.forward(50)
pen.left(90)
pen.forward(50)
# 关闭画布
canvas.exitonclick()
```
这段代码会在画布上绘制出一个蓝色的“L”,代表我的名字“李”字的第一个字母。你可以根据自己的名字和喜好修改代码,绘制出不同的图案。
### 回答2:
使用Python语言的turtle库,可以很轻松地写出自己的名字。接下来,就让我们一起来实现吧!
首先,我们需要将turtle库导入进来。如果你还没有安装turtle库,那么可以通过以下命令进行安装:
pip install turtle
在导入库之后,我们需要使用turtle库中的pen方法来控制乌龟的移动。在这个例子里,我们就要实现用turtle画出名字的字符。
首先,我们要来画出一个字母A。由于A的形状比较特殊,所以我们需要画出两条斜线和一条横线。代码如下:
```python
import turtle
# 初始化画布
turtle.setup(width=600, height=400)
# 创建一个进行绘画的海龟实例,并将画笔抬起来(不用绘制时要抬起画笔)
turtle.hideturtle()
turtle.penup()
# 将画笔移动到合适的位置
turtle.goto(-200, 0)
# 将画笔放下,开始绘制
turtle.pendown()
turtle.forward(100)
turtle.right(120)
turtle.forward(100)
turtle.right(120)
turtle.forward(100)
turtle.right(180)
turtle.forward(50)
turtle.right(60)
turtle.forward(50)
# 显示绘画结果
turtle.done()
```
运行这段代码,就可以看到屏幕上画出了一个字母A。接下来,我们可以按照同样的方式,画出自己名字的所有字符。
最终,我们可以把所有字符画在一起,代码如下:
```python
import turtle
def draw_A():
turtle.pendown()
turtle.forward(100)
turtle.right(120)
turtle.forward(100)
turtle.right(120)
turtle.forward(100)
turtle.right(180)
turtle.forward(50)
turtle.right(60)
turtle.forward(50)
def draw_R():
turtle.pendown()
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(50)
turtle.right(45)
turtle.forward(70)
turtle.right(45)
turtle.forward(50)
turtle.right(45)
turtle.forward(70)
turtle.right(45)
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
def draw_O():
turtle.pendown()
turtle.circle(50)
def draw_N():
turtle.penup()
turtle.right(180)
turtle.forward(25)
turtle.right(45)
turtle.pendown()
turtle.forward(70)
turtle.right(135)
turtle.forward(100)
turtle.right(135)
turtle.forward(70)
def draw_G():
turtle.pendown()
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(30)
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(30)
turtle.right(180)
turtle.forward(30)
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(70)
def draw_I():
turtle.pendown()
turtle.right(90)
turtle.forward(100)
turtle.penup()
turtle.right(180)
turtle.forward(50)
turtle.right(90)
turtle.pendown()
turtle.forward(100)
def draw_E():
turtle.pendown()
turtle.right(90)
turtle.forward(100)
turtle.right(180)
turtle.forward(100)
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
turtle.right(180)
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
turtle.right(90)
turtle.forward(50)
# 初始化画布
turtle.setup(width=600, height=400)
# 创建一个进行绘画的海龟实例,并将画笔抬起来(不用绘制时要抬起画笔)
turtle.hideturtle()
turtle.penup()
# 将画笔移动到合适的位置
turtle.goto(-250, 0)
# 画名字的每个字符
draw_A()
turtle.penup()
turtle.forward(50)
turtle.pendown()
draw_R()
turtle.penup()
turtle.forward(50)
turtle.pendown()
draw_O()
turtle.penup()
turtle.forward(50)
turtle.pendown()
draw_N()
turtle.penup()
turtle.forward(50)
turtle.pendown()
draw_G()
turtle.penup()
turtle.forward(80)
turtle.pendown()
draw_I()
turtle.penup()
turtle.forward(50)
turtle.pendown()
draw_E()
# 显示绘画结果
turtle.done()
```
运行这段代码,你就可以在屏幕上看到你的名字了!当然,你可以根据需要,调整字符的大小和位置,让它们更加漂亮。
### 回答3:
turtle作为Python中的绘图模块,它可以让我们通过代码来实现绘制图像,其中也包括了文字的绘制。下面我就来演示如何使用turtle绘制自己的名字。
首先,我们需要导入turtle模块,并且创建一个画布:
```
import turtle
canvas = turtle.Screen()
canvas.bgcolor('white')
canvas.title('Draw my name!')
```
其中,`bgcolor`和`title`分别设置了画布的背景颜色和标题。接着,我们需要创建一个turtle对象,并设置它的线条颜色和尺寸:
```
t = turtle.Turtle()
t.color('blue')
t.pensize(3)
```
接着,我们需要通过turtle绘制每个字母。以我的名字"JAMA"为例,我会先写出每个字母对应的绘制步骤:
- J:先向上画一条长60的竖线,然后向右下方画一条长度为80,角度为135的斜线,最后向左下方画一条长度为160,角度为225的斜线。
- A:先向上画一条长度为30的斜线,然后再向右下方画一条长度为60,角度为135的斜线,再向左下方画一条长度为60,角度为225的斜线,最后再从左上方向下画一条长度为30的竖线。
- M:先向上画一条长度为90的斜线,然后向右下方画一条长度为90,角度为135的斜线,再向左下方画一条长度为90,角度为225的斜线,最后再向上画一条长度为90的斜线。
- A:同上
根据上述绘制步骤,我们可以使用turtle来实现绘制。以下是完整的代码:
```
import turtle
canvas = turtle.Screen()
canvas.bgcolor('white')
canvas.title('Draw my name!')
t = turtle.Turtle()
t.color('blue')
t.pensize(3)
# 绘制J
t.penup()
t.goto(-200, 0)
t.pendown()
t.setheading(90)
t.forward(60)
t.setheading(315)
t.forward(80)
t.setheading(225)
t.forward(160)
# 绘制A
t.penup()
t.goto(-135, 0)
t.pendown()
t.setheading(90)
t.forward(30)
t.setheading(315)
t.forward(60)
t.setheading(225)
t.forward(60)
t.setheading(270)
t.forward(30)
# 绘制M
t.penup()
t.goto(-50, 0)
t.pendown()
t.setheading(90)
t.forward(90)
t.setheading(315)
t.forward(90)
t.setheading(225)
t.forward(90)
t.setheading(90)
t.forward(90)
# 绘制A
t.penup()
t.goto(115, 0)
t.pendown()
t.setheading(90)
t.forward(30)
t.setheading(315)
t.forward(60)
t.setheading(225)
t.forward(60)
t.setheading(270)
t.forward(30)
turtle.done()
```
最后,我们使用`done()`函数来实现绘图的展示。当程序运行时,窗口中应该会出现绘制好的自己名字的图案。同时,我们可以通过调整上述代码中的坐标和角度来实现自定义的自己名字绘制。
相关推荐
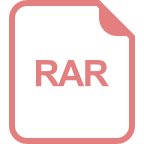














