请用Python写一段代码来利用卡尔曼滤波器检测视频内容中变化最大的50张帧并保存
时间: 2023-08-31 15:30:36 浏览: 163
### 回答1:
import cv2
import numpy as np
# Read the video
cap = cv2.VideoCapture('video.avi')
# Frame size
frame_width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
frame_height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# Kalman Filter
kalman = cv2.KalmanFilter(4,2)
# Defining the initial transition matrix, state transition matrix and process noise covariance matrix
kalman.transitionMatrix = np.array([[1,0,1,0],[0,1,0,1],[0,0,1,0],[0,0,0,1]])
kalman.measurementMatrix = np.array([[1,0,0,0],[0,1,0,0]])
kalman.processNoiseCov = np.array([[1,0,0,0],[0,1,0,0],[0,0,1,0],[0,0,0,1]]) * 0.03
# Initializing the frames
prev_frame = np.zeros((frame_height,frame_width,3), np.uint8)
curr_frame = np.zeros((frame_height,frame_width,3), np.uint8)
# Initializing the list of frames with highest changes
max_change_frames = []
while(cap.isOpened()):
# Reading the frames
ret, curr_frame = cap.read()
# Converting to grayscale
prev_gray = cv2.cvtColor(prev_frame, cv2.COLOR_BGR2GRAY)
curr_gray = cv2.cvtColor(curr_frame, cv2.COLOR_BGR2GRAY)
# Calculating the absolute difference
diff = cv2.absdiff(prev_gray, curr_gray)
# Blurring the image
blur = cv2.GaussianBlur(diff, (5,5), 0)
# Thresholding the image
_, thresh = cv2.threshold(blur, 20, 255, cv2.THRESH_BINARY)
# Finding contours
contours, _ = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# Iterating over contours and drawing a bounding box
for c in contours:
(x, y, w, h) = cv2.boundingRect(c)
# Calculating the Kalman Filter
state = np.array([[x+w/2.],[y+h/2.],[0.],[0.]])
kalman.correct(state)
state = kalman.predict()
# Finding the maximum change
change = abs(state[0][0] - (x+w/2.)) + abs(state[1][0] - (y+h/2.))
# Storing the frames with maximum changes
if change > 10:
max_change_frames.append(curr_frame)
# Storing the current frame
prev_frame = curr_frame.copy()
# Breaking the loop when 50 frames have been stored
if len(max_change_frames) == 50:
break
# Writing the frames to disk
for i in range(len(max_change_frames)):
frame_name = "frame_" + str(i) + ".jpg"
cv2.imwrite(frame_name, max_change_frames[i])
# Releasing the video capture
cap.release()
### 回答2:
首先,需要导入所需要的库:cv2(用于读取和处理视频帧)、numpy(用于处理数值计算)、matplotlib(用于绘图)、os(用于保存图片)和KalmanFilter(用于卡尔曼滤波器)。
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
import os
from filterpy.kalman import KalmanFilter
```
接下来,我们需要定义一个函数来计算图像的变化度量,例如差异值的平方和。
```python
def compute_frame_change(frame):
gray_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
return np.sum((gray_frame.astype(np.float32) - prev_frame.astype(np.float32))**2)
```
然后,我们需要打开视频文件并获取帧数和宽高。
```python
video_file = 'your_video_file_path' # 替换为你的视频文件路径
cap = cv2.VideoCapture(video_file)
num_frames = int(cap.get(cv2.CAP_PROP_FRAME_COUNT))
width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
```
接下来,我们初始化卡尔曼滤波器。
```python
kalman_filter = KalmanFilter(dim_x=2, dim_z=1)
kalman_filter.x = np.array([0., 1.])
kalman_filter.F = np.array([[1., 1.], [0., 1.]])
kalman_filter.H = np.array([[1., 0.]])
kalman_filter.P *= 1000
kalman_filter.R = 0.01
```
然后,我们开始循环读取每一帧并计算变化度量。我们可以用卡尔曼滤波器获得平滑的变化度量值。
```python
max_changes = []
for i in range(num_frames):
ret, frame = cap.read()
if not ret:
break
change = compute_frame_change(frame)
max_change = kalman_filter.update(change)
max_changes.append(max_change)
```
接下来,我们根据变化度量值的大小获取最大变化的50张帧。
```python
indices = np.argsort(max_changes)[-50:]
```
最后,我们将选定的50张帧保存到指定的文件夹中。
```python
output_folder = 'your_output_folder_path' # 替换为你的输出文件夹路径
os.makedirs(output_folder, exist_ok=True)
for i in indices:
cap.set(cv2.CAP_PROP_POS_FRAMES, i)
ret, frame = cap.read()
if not ret:
break
cv2.imwrite(os.path.join(output_folder, f'frame_{i}.jpg'), frame)
```
这段代码将检测视频内容中变化最大的50张帧,并将其保存在指定的文件夹中。请注意,你需要替换`'your_video_file_path'`和`'your_output_folder_path'`为你的实际路径。
阅读全文
相关推荐
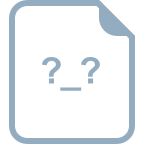


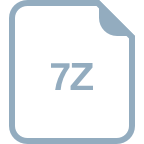
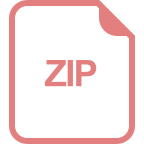
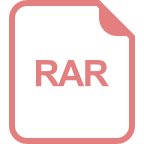
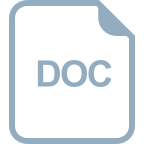
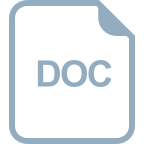
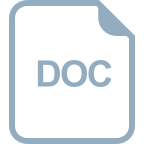



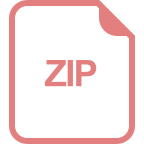
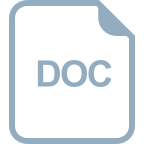
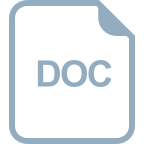

