springboot实现excel数据导入到mysql
时间: 2023-11-26 11:06:39 浏览: 41
要实现Excel数据导入到MySQL数据库,可以采用以下步骤:
1. 在Spring Boot项目中添加Apache POI和MySQL数据库的依赖。
2. 创建一个包含Excel数据的POJO类,例如Student类,用于存储Excel中的每一行数据。
3. 创建一个Excel文件上传的Controller类,实现文件上传功能,读取Excel数据并将数据存储在List<Student>中。
4. 创建一个Service类,实现将List<Student>中的数据插入到MySQL数据库的功能。
5. 在Service类中使用JDBC或MyBatis等框架,连接 MySQL 数据库,将Excel数据插入到数据库。
下面是一个简单的实现:
1. 添加依赖
在pom.xml中添加Apache POI和MySQL数据库的依赖:
```
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.1</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.1</version>
</dependency>
<!-- MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.22</version>
</dependency>
```
2. 创建POJO类
创建一个Student类,用于存储Excel中的每一行数据:
```java
public class Student {
private String name;
private int age;
private String gender;
// 省略getter和setter方法
}
```
3. 创建Controller类
创建一个Excel文件上传的Controller类,实现文件上传功能,读取Excel数据并将数据存储在List<Student>中:
```java
@RestController
@RequestMapping("/api/excel")
public class ExcelController {
@PostMapping("/upload")
public String upload(@RequestParam("file") MultipartFile file) {
try {
List<Student> students = new ArrayList<>();
// 读取Excel文件
Workbook workbook = WorkbookFactory.create(file.getInputStream());
Sheet sheet = workbook.getSheetAt(0);
// 遍历每一行数据
for (Row row : sheet) {
Student student = new Student();
student.setName(row.getCell(0).getStringCellValue());
student.setAge((int)row.getCell(1).getNumericCellValue());
student.setGender(row.getCell(2).getStringCellValue());
students.add(student);
}
// 调用Service类将数据插入到MySQL数据库
studentService.insertStudents(students);
return "上传成功!";
} catch (Exception e) {
e.printStackTrace();
return "上传失败!";
}
}
}
```
4. 创建Service类
创建一个Service类,实现将List<Student>中的数据插入到MySQL数据库的功能:
```java
@Service
public class StudentService {
@Autowired
private JdbcTemplate jdbcTemplate;
public void insertStudents(List<Student> students) {
String sql = "INSERT INTO student(name, age, gender) VALUES (?, ?, ?)";
for (Student student : students) {
jdbcTemplate.update(sql, student.getName(), student.getAge(), student.getGender());
}
}
}
```
5. 测试
启动Spring Boot应用程序,并使用Postman或其他工具向/upload端点上传Excel文件,可以看到数据已成功插入到MySQL数据库中。
以上就是Spring Boot实现Excel数据导入到MySQL的简单实现。
相关推荐
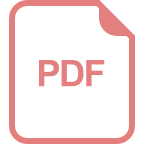
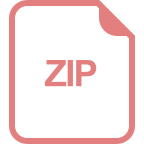
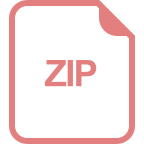










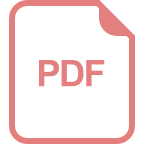
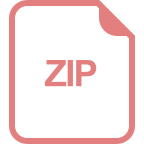
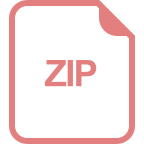
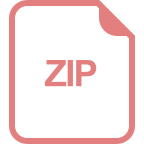