threejs uniapp
时间: 2023-09-08 21:10:30 浏览: 43
Three.js is a popular JavaScript library for creating 3D graphics and animations in web browsers. Uniapp is a framework for developing cross-platform mobile applications using Vue.js.
To use Three.js in a Uniapp project, you can include the Three.js library in your project and use it to create 3D graphics and animations in your Vue components. Here are the steps to get started:
1. Install Three.js using npm or include it in your project using a script tag.
2. Create a new Vue component for your 3D scene.
3. In the component's mounted hook, create a new Three.js scene, camera, renderer, and other necessary objects.
4. Add your 3D objects to the scene and position them as desired.
5. In the component's update loop, update the position, rotation, and other properties of your 3D objects to animate them.
6. Render the scene using the Three.js renderer.
Here is a basic example of a Three.js scene in a Vue component:
```
<template>
<div ref="container"></div>
</template>
<script>
import * as THREE from 'three';
export default {
mounted() {
// create the Three.js scene, camera, and renderer
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setSize(window.innerWidth, window.innerHeight);
this.$refs.container.appendChild(renderer.domElement);
// add a cube to the scene
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
// position the camera and cube
camera.position.z = 5;
cube.position.x = -2;
// animate the cube
const animate = () => {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
};
animate();
},
};
</script>
```
This is just a basic example, and there are many more advanced techniques you can use with Three.js in a Uniapp project. You can find more information and examples on the Three.js website and in the Uniapp documentation.
相关推荐
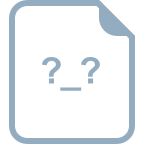














