python贪吃蛇自动寻路
时间: 2023-11-01 10:57:35 浏览: 40
你想问关于Python贪吃蛇自动寻路的问题,是吗?那么,你可以使用一些算法来实现贪吃蛇的自动寻路。其中,最常见的算法是A*(A-star)算法。
A*算法是一种启发式搜索算法,用于在图中找到最短路径。在贪吃蛇的情景中,你可以将地图看作是一个二维的网格,每个位置上可以是空白、食物或者蛇的身体。贪吃蛇的目标是从当前位置移动到食物所在的位置。
下面是一个简单的示例代码,展示了如何使用A*算法实现贪吃蛇的自动寻路:
```python
import heapq
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def astar(start, target, obstacles):
open_set = []
closed_set = set()
heapq.heappush(open_set, (0, start))
while open_set:
current_cost, current_node = heapq.heappop(open_set)
if current_node == target:
return True
closed_set.add(current_node)
for neighbor in neighbors(current_node):
if neighbor in closed_set or neighbor in obstacles:
continue
new_cost = current_cost + 1
heapq.heappush(open_set, (new_cost + heuristic(neighbor, target), neighbor))
return False
def neighbors(node):
x, y = node
return [(x-1, y), (x+1, y), (x, y-1), (x, y+1)]
# 示例用法
start = (0, 0)
target = (9, 9)
obstacles = [(1, 1), (1, 2), (2, 2), (2, 3), (3, 3), (4, 3), (5, 3)]
is_path_exists = astar(start, target, obstacles)
print(is_path_exists)
```
这个示例代码中,`start`代表贪吃蛇的当前位置,`target`代表食物的位置,`obstacles`代表蛇的身体或其他障碍物的位置。`astar`函数使用A*算法来判断是否存在从起点到终点的路径,并返回相应的结果。
请注意,以上代码只是一个简单示例,你可能需要根据实际情况进行适当的修改和扩展。希望对你有所帮助!如果你有任何进一步的问题,请随时提问。
相关推荐
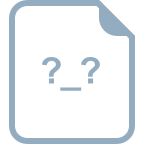














