ffmpeg对音频的byte[]编码,并返回编码后的byte[]
时间: 2023-11-13 15:03:48 浏览: 154
可以使用ffmpeg的libavcodec库来对音频进行编码,具体步骤如下:
1. 初始化ffmpeg库
```c
avcodec_register_all();
```
2. 打开编码器
```c
AVCodec *codec = avcodec_find_encoder(AV_CODEC_ID_MP3); // 指定编码器为MP3
AVCodecContext *codec_ctx = avcodec_alloc_context3(codec);
avcodec_open2(codec_ctx, codec, NULL);
```
3. 准备输入数据
输入数据需要以AVFrame的形式提供给编码器,可以参考以下代码:
```c
AVFrame *frame = av_frame_alloc();
frame->nb_samples = nb_samples; // 采样数
frame->format = codec_ctx->sample_fmt; // 样本格式
frame->channel_layout = codec_ctx->channel_layout; // 声道布局
av_frame_get_buffer(frame, 0);
// 将音频数据写入AVFrame中
memcpy(frame->data[0], audio_data, audio_size);
```
4. 编码音频数据
```c
AVPacket pkt;
av_init_packet(&pkt);
pkt.data = NULL;
pkt.size = 0;
int ret = avcodec_send_frame(codec_ctx, frame);
if (ret < 0) {
// 发送音频数据失败
}
ret = avcodec_receive_packet(codec_ctx, &pkt);
if (ret < 0) {
// 编码音频数据失败
}
// 此时pkt.data中存储的就是编码后的音频数据
uint8_t *encoded_data = pkt.data;
int encoded_size = pkt.size;
```
5. 释放资源
```c
av_packet_unref(&pkt);
av_frame_free(&frame);
avcodec_close(codec_ctx);
avcodec_free_context(&codec_ctx);
```
以上是对音频进行编码的基本流程,具体实现需要根据自己的需求进行调整。
阅读全文
相关推荐
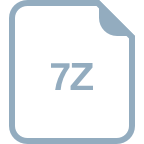


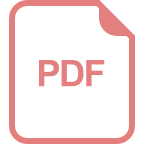
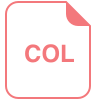
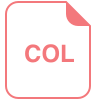
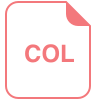
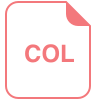
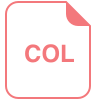
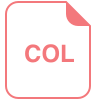






