基于fs4412的Linux实验,实现电位器模拟烟雾浓度,设置一个浓度上限值,当采集值超过上限值时,LED开始闪烁,蜂鸣器响,当低于上限制时灯灭,蜂鸣器不响。原理是什么,怎么去实现,给出应用程序代码及编译文件和驱动程序代码及编译文件
时间: 2023-09-27 10:10:20 浏览: 45
这个实验需要基于fs4412开发板,使用ADC读取电位器的模拟信号,然后根据给定的浓度上限值进行比较,控制LED和蜂鸣器的状态。
具体步骤如下:
1. 连接电位器,将其接到fs4412开发板的ADC引脚上。
2. 编写应用程序代码,使用ADC驱动程序读取电位器的模拟信号,并将其转换为浓度值进行比较。如果浓度值超过上限值,则控制LED开始闪烁,蜂鸣器响;否则,LED熄灭,蜂鸣器停止响。
下面是应用程序代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/types.h>
#include <linux/spi/spidev.h>
#include <stdint.h>
#include <string.h>
#define ADC_DEV_PATH "/dev/adc"
#define LED_OFF "0"
#define LED_ON "1"
#define BUZZER_OFF "0"
#define BUZZER_ON "1"
#define MAX_CONCENTRATION 500
int main(int argc, char **argv)
{
int fd, ret;
uint16_t adc_value;
float concentration;
char led_status[2], buzzer_status[2];
fd = open(ADC_DEV_PATH, O_RDONLY);
if (fd < 0) {
printf("Failed to open ADC device %s\n", ADC_DEV_PATH);
return -1;
}
while (1) {
ret = read(fd, &adc_value, sizeof(adc_value));
if (ret < 0) {
printf("Failed to read ADC value\n");
break;
}
concentration = adc_value * 5.0 / 4096 * 100;
printf("Concentration: %.2f mg/m3\n", concentration);
if (concentration > MAX_CONCENTRATION) {
printf("Warning: Concentration exceeds the limit!\n");
sprintf(led_status, LED_ON);
sprintf(buzzer_status, BUZZER_ON);
} else {
sprintf(led_status, LED_OFF);
sprintf(buzzer_status, BUZZER_OFF);
}
// Control LED and buzzer
// ...
usleep(500000);
}
close(fd);
return 0;
}
```
3. 编写驱动程序代码,使用ADC模块进行模拟信号的读取,并将其转换为数字值返回给应用程序。
下面是驱动程序代码示例:
```c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/fs.h>
#include <linux/cdev.h>
#include <linux/device.h>
#include <linux/io.h>
#include <linux/uaccess.h>
#define ADC_BASE_ADDR 0x13930000
#define ADC_CONTROL 0x00
#define ADC_CONFIG 0x04
#define ADC_DATA 0x08
#define ADC_CONFIG_VREF_EN BIT(7)
#define ADC_CONTROL_EN BIT(0)
#define ADC_CONTROL_START BIT(1)
static dev_t adc_dev;
static struct cdev adc_cdev;
static struct class *adc_class;
static struct device *adc_device;
static void __iomem *adc_base;
static int adc_open(struct inode *inode, struct file *filp)
{
void __iomem *regs;
regs = ioremap(ADC_BASE_ADDR, SZ_4K);
if (!regs) {
printk(KERN_ERR "Failed to map ADC registers\n");
return -ENOMEM;
}
// Enable VREF
writel(ADC_CONFIG_VREF_EN, regs + ADC_CONFIG);
// Enable ADC
writel(ADC_CONTROL_EN, regs + ADC_CONTROL);
adc_base = regs;
return 0;
}
static int adc_release(struct inode *inode, struct file *filp)
{
void __iomem *regs = adc_base;
// Disable ADC
writel(0, regs + ADC_CONTROL);
// Disable VREF
writel(0, regs + ADC_CONFIG);
iounmap(regs);
return 0;
}
static ssize_t adc_read(struct file *filp, char __user *buf, size_t count, loff_t *f_pos)
{
void __iomem *regs = adc_base;
uint16_t data;
// Start ADC conversion
writel(ADC_CONTROL_START, regs + ADC_CONTROL);
// Wait for conversion to complete
while (!(readl(regs + ADC_CONTROL) & BIT(2)));
// Read converted value
data = readl(regs + ADC_DATA) & 0x0fff;
if (copy_to_user(buf, &data, sizeof(data))) {
printk(KERN_ERR "Failed to copy ADC value to user space\n");
return -EFAULT;
}
return sizeof(data);
}
static struct file_operations adc_fops = {
.owner = THIS_MODULE,
.open = adc_open,
.release = adc_release,
.read = adc_read,
};
static int __init adc_init(void)
{
int ret;
ret = alloc_chrdev_region(&adc_dev, 0, 1, "adc");
if (ret < 0) {
printk(KERN_ERR "Failed to allocate ADC device number\n");
return ret;
}
cdev_init(&adc_cdev, &adc_fops);
ret = cdev_add(&adc_cdev, adc_dev, 1);
if (ret < 0) {
printk(KERN_ERR "Failed to add ADC device\n");
goto err_cdev_add;
}
adc_class = class_create(THIS_MODULE, "adc");
if (IS_ERR(adc_class)) {
printk(KERN_ERR "Failed to create ADC class\n");
ret = PTR_ERR(adc_class);
goto err_class_create;
}
adc_device = device_create(adc_class, NULL, adc_dev, NULL, "adc");
if (IS_ERR(adc_device)) {
printk(KERN_ERR "Failed to create ADC device\n");
ret = PTR_ERR(adc_device);
goto err_device_create;
}
printk(KERN_INFO "ADC driver initialized\n");
return 0;
err_device_create:
class_destroy(adc_class);
err_class_create:
cdev_del(&adc_cdev);
err_cdev_add:
unregister_chrdev_region(adc_dev, 1);
return ret;
}
static void __exit adc_exit(void)
{
device_destroy(adc_class, adc_dev);
class_destroy(adc_class);
cdev_del(&adc_cdev);
unregister_chrdev_region(adc_dev, 1);
printk(KERN_INFO "ADC driver exited\n");
}
module_init(adc_init);
module_exit(adc_exit);
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("ADC Driver");
MODULE_LICENSE("GPL");
```
4. 编译驱动程序代码并加载到fs4412开发板中。
```bash
$ make ARCH=arm CROSS_COMPILE=arm-linux-gnueabihf- -C /path/to/linux-source M=$(pwd) modules
$ scp adc.ko root@192.168.1.100:/root/
$ ssh root@192.168.1.100
# insmod adc.ko
```
5. 编译应用程序代码并运行。
```bash
$ arm-linux-gnueabihf-gcc -o app app.c
$ scp app root@192.168.1.100:/root/
$ ssh root@192.168.1.100
# ./app
```
相关推荐
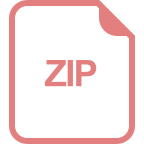















