pycharm爬取网页数据保存mysql中
时间: 2023-12-29 18:13:39 浏览: 43
好的,这个问题是关于编程和技术的,我可以给您提供一些指导和建议。通常,您可以使用Python编写爬虫程序,使用PyCharm作为开发工具进行编写和调试。对于将爬取到的数据保存到MySQL中,您需要先安装Python的MySQL驱动包,然后使用Python的数据库API(如MySQLdb)进行连接、查询和插入操作,最后将爬取到的数据以合适的形式插入到MySQL数据库中。如果您有具体的问题,可以提出来,我会尽力为您解答。
相关问题
pycharm爬取网上数据存入mysql的代码
以下是一个简单的 Python 代码示例,演示如何使用 PyCharm 爬取网上数据并将其存储到 MySQL 数据库中:
首先,需要安装 `requests` 和 `beautifulsoup4` 这两个库,以便从网页上获取数据并进行解析:
```
pip install requests
pip install beautifulsoup4
```
然后,需要安装 MySQL Connector 驱动程序:
```
pip install mysql-connector-python
```
接下来,可以使用以下代码从网页上获取数据并将其存储到 MySQL 数据库中:
```python
import requests
from bs4 import BeautifulSoup
import mysql.connector
# 连接 MySQL 数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
# 获取网页内容
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 解析数据
data = []
for item in soup.find_all('div', class_='item'):
title = item.find('h2').text.strip()
description = item.find('p').text.strip()
data.append((title, description))
# 将数据存储到 MySQL 数据库中
mycursor = mydb.cursor()
sql = "INSERT INTO items (title, description) VALUES (%s, %s)"
mycursor.executemany(sql, data)
mydb.commit()
```
在以上代码中,需要将 `yourusername`、`yourpassword` 和 `mydatabase` 替换为您自己的 MySQL 数据库的用户名、密码和数据库名称。同时,需要将 `https://example.com` 替换为您要爬取数据的网址,并根据需要修改解析数据的代码。
以上代码只是一个简单示例,实际情况可能会更加复杂。如果您在使用 PyCharm 进行爬虫开发时遇到问题,可以查看 PyCharm 的文档和官方论坛,寻求帮助。
pycharm爬取网页数据
可以使用Python的requests库和BeautifulSoup库来爬取网页数据。以下是一个简单的代码示例:
```
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 获取页面标题
title = soup.title.text
print(title)
# 获取页面所有链接
links = []
for link in soup.find_all('a'):
links.append(link.get('href'))
print(links)
```
在上面的代码中,首先使用requests库发送HTTP请求并获取网页的响应结果,然后使用BeautifulSoup库解析HTML文档并提取所需的数据。在这个示例中,我们获取了页面的标题和所有链接。你可以根据自己的需求来提取其他数据。
相关推荐
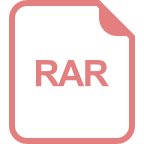
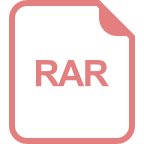
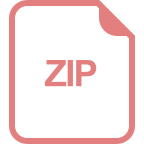
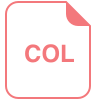
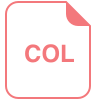
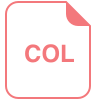









