用java语言将整数序列(4,5,7,2,1,3,6)中的整数依次插入到一棵空的平衡二叉树中,试构造相应的平衡二叉树
时间: 2024-06-13 19:08:44 浏览: 15
以下是Java语言将整数序列插入到平衡二叉树的代码实现:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
int[] nums = {4, 5, 7, 2, 1, 3, 6};
TreeNode root = null;
for (int num : nums) {
root = insert(root, num);
}
System.out.println("平衡二叉树的中序遍历结果为:");
inorderTraversal(root);
}
// 定义平衡二叉树的节点
static class TreeNode {
int val;
int height;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
this.height = 1;
}
}
// 计算节点高度
static int height(TreeNode node) {
return node == null ? 0 : node.height;
}
// 计算节点平衡因子
static int balanceFactor(TreeNode node) {
return node == null ? 0 : height(node.left) - height(node.right);
}
// 右旋操作
static TreeNode rightRotate(TreeNode y) {
TreeNode x = y.left;
TreeNode T3 = x.right;
x.right = y;
y.left = T3;
y.height = Math.max(height(y.left), height(y.right)) + 1;
x.height = Math.max(height(x.left), height(x.right)) + 1;
return x;
}
// 左旋操作
static TreeNode leftRotate(TreeNode x) {
TreeNode y = x.right;
TreeNode T2 = y.left;
y.left = x;
x.right = T2;
x.height = Math.max(height(x.left), height(x.right)) + 1;
y.height = Math.max(height(y.left), height(y.right)) + 1;
return y;
}
// 插入节点
static TreeNode insert(TreeNode node, int val) {
if (node == null) {
return new TreeNode(val);
}
if (val < node.val) {
node.left = insert(node.left, val);
} else if (val > node.val) {
node.right = insert(node.right, val);
} else {
return node;
}
node.height = Math.max(height(node.left), height(node.right)) + 1;
int balanceFactor = balanceFactor(node);
if (balanceFactor > 1 && val < node.left.val) {
return rightRotate(node);
}
if (balanceFactor < -1 && val > node.right.val) {
return leftRotate(node);
}
if (balanceFactor > 1 && val > node.left.val) {
node.left = leftRotate(node.left);
return rightRotate(node);
}
if (balanceFactor < -1 && val < node.right.val) {
node.right = rightRotate(node.right);
return leftRotate(node);
}
return node;
}
// 中序遍历
static void inorderTraversal(TreeNode node) {
if (node != null) {
inorderTraversal(node.left);
System.out.print(node.val + " ");
inorderTraversal(node.right);
}
}
}
```
相关推荐
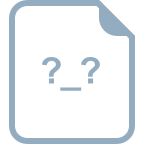














