python数据集划分
时间: 2025-01-05 07:17:53 浏览: 7
### 数据集划分方法
在Python中,数据集通常被划分为训练集、验证集和测试集来评估模型性能并防止过拟合。常用库`scikit-learn`提供了简单易用的功能来进行这种操作。
#### 使用Scikit-Learn进行随机划分
对于简单的二分类场景——即仅需区分训练集与测试集的情况,可利用`train_test_split()`函数完成快速分割:
```python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(
X, y, test_size=0.2, random_state=42)
```
此处参数解释如下:
- `X`: 特征矩阵;
- `y`: 标签向量;
- `test_size`: 测试样本占比,默认为0.25;
- `random_state`: 随机种子数,用于重现相同的结果[^1]。
当涉及到更复杂的三类划分时,则可以通过两次调用上述命令实现:
```python
# 第一次切分得到训练集+验证集 和 测试集
X_temp, X_test, y_temp, y_test = train_test_split(X, y, test_size=test_ratio, random_state=random_seed)
# 对剩余部分再次切割获得最终的训练集和验证集
X_train, X_val, y_train, y_val = train_test_split(X_temp, y_temp, test_size=val_ratio/(1-test_ratio), random_state=random_seed)
```
这里需要注意的是,在第二次分裂之前已经预留了一定量作为测试集合;因此实际分配给验证集的比例应当基于除去测试后的总量计算得出[^3]。
另外一种更为直观的方式是通过指定确切数量而非百分比来进行手动控制各子集中元素数目:
```python
def split_dataset(dataframe, ratios=[0.7, 0.15, 0.15]):
assert sum(ratios) == 1., "The provided ratio does not add up to one."
total_length = len(dataframe)
indices = list(range(total_length))
np.random.shuffle(indices)
start_index = 0
splits = []
for r in ratios[:-1]:
end_index = int(start_index + round(r * total_length))
subset_indices = sorted(indices[start_index:end_index])
splits.append(subset_indices)
start_index = end_index
last_subset_indices = sorted(indices[start_index:])
splits.append(last_subset_indices)
return tuple([dataframe.iloc[s].reset_index(drop=True) for s in splits])
df_train, df_valid, df_test = split_dataset(df, [0.6, 0.2, 0.2])
```
此自定义函数接受一个DataFrame对象以及期望的比例列表作为输入,并返回按顺序排列好的三个新表分别对应于训练集、验证集和测试集[^5]。
阅读全文
相关推荐














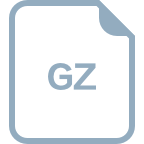

