使用pygame实现弹窗
时间: 2024-06-15 08:01:41 浏览: 254
Pygame是一个流行的Python游戏开发库,虽然它的主要目的是创建2D游戏,但它也可以用于创建简单的窗口和用户界面,包括弹窗(dialog boxes)或提示信息。在Pygame中,我们可以使用`pygame.display`模块的`surface`对象来创建和管理窗口,以及`pygame.event`模块来处理用户的交互,如鼠标点击。
以下是使用Pygame实现一个简单弹窗的基本步骤:
1. 导入所需的库:
```python
import pygame
from pygame.locals import *
```
2. 初始化Pygame:
```python
pygame.init()
```
3. 创建一个窗口(包含一个背景):
```python
screen = pygame.display.set_mode((800, 600))
background_color = (255, 255, 255) # 设置背景颜色
screen.fill(background_color)
```
4. 弹窗内容和样式:
```python
message = "这是一条弹窗消息"
font = pygame.font.SysFont('Arial', 36) # 选择字体和大小
text_surface = font.render(message, True, (0, 0, 0)) # 渲染文本
text_rect = text_surface.get_rect() # 获取文本矩形位置
text_rect.center = screen.get_rect().center # 将文本置于屏幕中心
```
5. 检查事件和显示窗口:
```python
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
# 当鼠标点击时,打开弹窗
screen.blit(text_surface, text_rect)
pygame.display.update()
# 在这里添加关闭弹窗的逻辑,例如添加另一个事件处理
# 更新屏幕
pygame.display.flip()
# 关闭Pygame
pygame.quit()
```
阅读全文
相关推荐

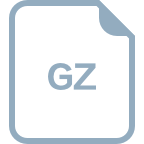
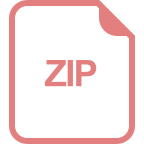
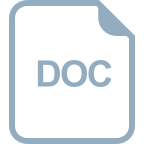
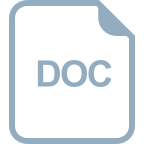

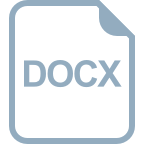
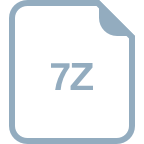
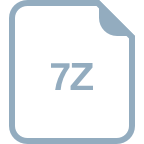
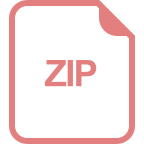