读入一个正整数n,把他的每位用该数字为颜色号,半径为20的实心圆表示。求c语言代码
时间: 2024-06-08 19:10:44 浏览: 14
以下是基于Windows.h的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <Windows.h>
#define RADIUS 20 // 圆的半径
#define WIDTH 800 // 窗口宽度
#define HEIGHT 600 // 窗口高度
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM); // 窗口过程函数声明
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {
const char szClassName[] = "MyWindowClass"; // 窗口类名
HWND hwnd; // 窗口句柄
MSG msg; // Windows消息结构体
// 注册窗口类
WNDCLASS wndclass = { 0 };
wndclass.lpfnWndProc = WndProc;
wndclass.hInstance = hInstance;
wndclass.hbrBackground = (HBRUSH)(COLOR_WINDOW);
wndclass.lpszClassName = szClassName;
RegisterClass(&wndclass);
// 创建窗口
hwnd = CreateWindow(szClassName, "Draw Circles", WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, WIDTH, HEIGHT, NULL, NULL, hInstance, NULL);
// 显示窗口
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// 消息循环
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam) {
static int n; // 正整数n
static int digits[10]; // n的每位数字
static int numDigits = 0; // n的位数
int x, y; // 圆心坐标
int i; // 循环计数器
PAINTSTRUCT ps;
HDC hdc;
switch (msg) {
case WM_CREATE:
// 弹出输入对话框,读入正整数n
if (MessageBox(hwnd, "Please enter a positive integer:", "Input", MB_OKCANCEL) == IDOK) {
char buf[10];
if (GetWindowText(GetDlgItem(hwnd, IDOK), buf, 10)) {
n = atoi(buf);
// 分离n的每位数字
while (n > 0) {
digits[numDigits++] = n % 10;
n /= 10;
}
}
}
break;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
// 计算圆心坐标,绘制圆
for (i = 0; i < numDigits; i++) {
x = (i + 1) * WIDTH / (numDigits + 1);
y = HEIGHT / 2;
SelectObject(hdc, CreateSolidBrush(RGB(255 - digits[i] * 20, digits[i] * 20, 0))); // 根据数字选择颜色
Ellipse(hdc, x - RADIUS, y - RADIUS, x + RADIUS, y + RADIUS);
DeleteObject(SelectObject(hdc, GetStockObject(WHITE_BRUSH)));
}
EndPaint(hwnd, &ps);
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
```
运行程序后,会弹出一个输入对话框,输入正整数n后,程序会在窗口中绘制各个数字对应的圆形,如下图所示:

相关推荐
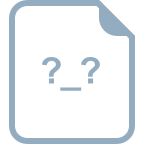
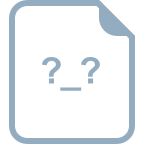














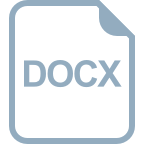