设计一个字符串类,实现该类的+,+=,>运算符重载
时间: 2024-03-03 13:49:21 浏览: 180
好的,以下是一个简单的字符串类的实现,其中重载了+、+=和>运算符:
```cpp
#include <cstring>
#include <iostream>
class String {
public:
// 默认构造函数
String() : data(nullptr), len(0) {}
// 构造函数,根据传入的字符串构造
String(const char* s) {
len = std::strlen(s);
data = new char[len + 1];
std::strcpy(data, s);
}
// 拷贝构造函数
String(const String& other) {
len = other.len;
data = new char[len + 1];
std::strcpy(data, other.data);
}
// 移动构造函数
String(String&& other) noexcept {
len = other.len;
data = other.data;
other.len = 0;
other.data = nullptr;
}
// 析构函数
~String() {
delete[] data;
}
// 重载+运算符
String operator+(const String& other) const {
String result;
result.len = len + other.len;
result.data = new char[result.len + 1];
std::strcpy(result.data, data);
std::strcat(result.data, other.data);
return result;
}
// 重载+=运算符
String& operator+=(const String& other) {
len += other.len;
char* newData = new char[len + 1];
std::strcpy(newData, data);
std::strcat(newData, other.data);
delete[] data;
data = newData;
return *this;
}
// 重载>运算符
bool operator>(const String& other) const {
return std::strcmp(data, other.data) > 0;
}
// 重载赋值运算符
String& operator=(const String& other) {
if (this != &other) {
delete[] data;
len = other.len;
data = new char[len + 1];
std::strcpy(data, other.data);
}
return *this;
}
// 重载移动赋值运算符
String& operator=(String&& other) noexcept {
if (this != &other) {
delete[] data;
len = other.len;
data = other.data;
other.len = 0;
other.data = nullptr;
}
return *this;
}
// 获取字符串长度
size_t length() const {
return len;
}
// 获取字符串数据
const char* c_str() const {
return data;
}
private:
char* data;
size_t len;
};
// 重载输出运算符
std::ostream& operator<<(std::ostream& out, const String& s) {
out << s.c_str();
return out;
}
int main() {
String s1("hello");
String s2("world");
String s3 = s1 + s2;
std::cout << s3 << std::endl;
s1 += s2;
std::cout << s1 << std::endl;
std::cout << (s3 > s1) << std::endl;
return 0;
}
```
在上面的代码中,我们实现了一个简单的字符串类`String`,它包含了一个指向字符数组的指针`data`和一个表示字符串长度的整数`len`。在构造函数、析构函数、拷贝构造函数和移动构造函数中,我们使用了`new`和`delete`来动态分配和释放内存。在重载+和+=运算符中,我们使用了`strcat`和`strcpy`等字符串操作函数来实现字符串的拼接。在重载>运算符中,我们使用了`strcmp`函数来比较两个字符串的大小。最后,我们还重载了输出运算符`<<`,使得我们可以方便地输出一个字符串对象。
阅读全文
相关推荐
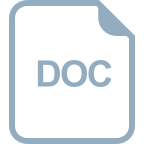
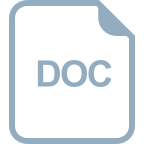
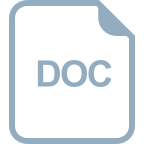

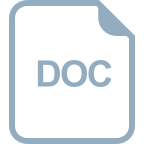




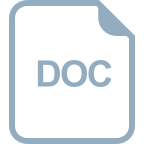
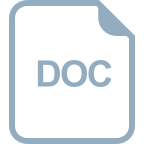


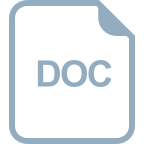
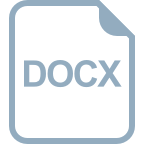