如何使用正则表达式在给定字符串的某个数字后添加一个空格?
时间: 2024-11-27 12:11:44 浏览: 0
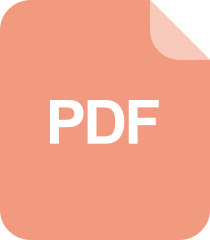
利用正则表达式判断一个给定的字符是否是回文
可以使用正则表达式的替换功能来实现这个需求。假设你想在字符串中的每个数字后面添加一个空格,你可以编写一个模式匹配规则,找到所有的数字,并在其后面插入一个`\s`(表示空格)。下面是一个简单的Python示例:
```python
import re
def add_space_after_digits(input_string):
pattern = r"(\d)" # 匹配一个数字
replacement = r"\1 " # 将匹配到的数字后面加上一个空格
result = re.sub(pattern, replacement, input_string)
return result
# 测试
input_str = "Hello123World456"
new_str = add_space_after_digits(input_str)
print(new_str) # 输出: Hello123 World456
```
在这个例子中,`re.sub()`函数会搜索输入字符串并用新字符串替换所有匹配到的数字,每找到一个数字就在其后加一个空格。
阅读全文
相关推荐
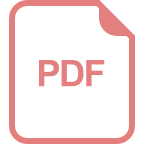
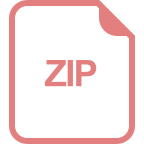
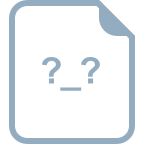
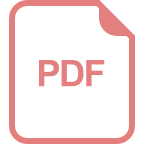
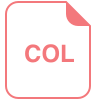
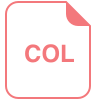
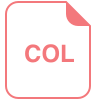
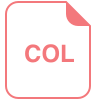








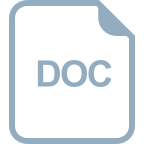
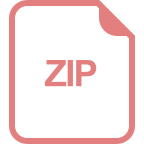