byte[] bytes = new byte[(int) files[0].length()]; FileInputStream fileInputStream = new FileInputStream(files[0]); fileInputStream.read(bytes); fileInputStream.close(); String fileDataString = String.valueOf(ByteString.of(bytes)); 换成base64
时间: 2024-03-06 21:51:25 浏览: 15
可以使用Java自带的Base64类将byte数组转换成Base64编码的字符串。修改代码如下所示:
```
byte[] bytes = new byte[(int) files[0].length()];
FileInputStream fileInputStream = new FileInputStream(files[0]);
fileInputStream.read(bytes);
fileInputStream.close();
String fileDataString = Base64.getEncoder().encodeToString(bytes);
```
其中,`Base64.getEncoder().encodeToString(bytes)`方法将byte数组转换成Base64编码的字符串。
相关问题
ToBServiceHelper.getInstance().getServiceBinder().pbsCapture(); File dir = new File(Environment.getExternalStorageDirectory().getAbsolutePath()+"/Pictures/Screenshots"); // 要读取的目录 File[] files = dir.listFiles(); // 获取目录下的所有文件 // 使用最后修改时间来排序文件数组 Arrays.sort(files, new Comparator<File>() { public int compare(File f1, File f2) { return Long.compare(f2.lastModified(), f1.lastModified()); } }); Log.i("Unity", "总共有多少文件: "+files.length); Log.i("Unity", "最后一个文件叫: "+files[0].getName()); byte[] bytes = new byte[(int) files[0].length()]; FileInputStream fileInputStream = new FileInputStream(files[0]); fileInputStream.read(bytes); fileInputStream.close(); String fileDataString = Base64.getEncoder().encodeToString(bytes); /** * 发送截屏数据 */ JSONObject jsondata =new JSONObject(); jsondata.put("opcode",OpCode.Send_Screenshot.getValue()); JSONObject content =new JSONObject(); content.put("pic",fileDataString); jsondata.put("content",content); SocketClientService.sendMessage(jsondata.toString()); files[0].delete();有报错 Attempt to get length of null array
这个报错可能是因为 `dir.listFiles()` 返回了空数组,导致 `files` 为空。你可以在使用 `files` 之前先判断一下它是否为空,例如:
```
if (files != null && files.length > 0) {
// 使用最后修改时间来排序文件数组
Arrays.sort(files, new Comparator<File>() {
public int compare(File f1, File f2) {
return Long.compare(f2.lastModified(), f1.lastModified());
}
});
Log.i("Unity", "总共有多少文件: "+files.length);
Log.i("Unity", "最后一个文件叫: "+files[0].getName());
byte[] bytes = new byte[(int) files[0].length()];
FileInputStream fileInputStream = new FileInputStream(files[0]);
fileInputStream.read(bytes);
fileInputStream.close();
String fileDataString = Base64.getEncoder().encodeToString(bytes);
/**
* 发送截屏数据
*/
JSONObject jsondata =new JSONObject();
jsondata.put("opcode",OpCode.Send_Screenshot.getValue());
JSONObject content =new JSONObject();
content.put("pic",fileDataString);
jsondata.put("content",content);
SocketClientService.sendMessage(jsondata.toString());
files[0].delete();
} else {
Log.i("Unity", "目录下没有文件!");
}
```
java代码如何将List<File> files的多文件集合压缩为zip格式并返回这个zip文件
您好,以下是Java代码将List<File> files的多文件集合压缩为zip格式并返回这个zip文件的实现方式:
```java
import java.io.*;
import java.util.*;
import java.util.zip.*;
public class ZipFiles {
public File zipFiles(List<File> files) throws IOException {
File zipFile = File.createTempFile("temp", ".zip");
FileOutputStream fos = new FileOutputStream(zipFile);
ZipOutputStream zos = new ZipOutputStream(fos);
for (File file : files) {
FileInputStream fis = new FileInputStream(file);
String entryName = file.getName();
ZipEntry zipEntry = new ZipEntry(entryName);
zos.putNextEntry(zipEntry);
byte[] bytes = new byte[1024];
int length;
while ((length = fis.read(bytes)) >= 0) {
zos.write(bytes, 0, length);
}
zos.closeEntry();
fis.close();
}
zos.close();
fos.close();
return zipFile;
}
}
```
实现过程中,我们首先创建一个 ZipOutputStream 对象,通过 putNextEntry 方法设置下一个将要写入的 ZipEntry 对象。对于每个要压缩的文件,我们使用 FileInputStream 读取文件内容,并将其写入 ZipEntry 中。最后,我们关闭 ZipEntry 对象、文件输入流和 ZipOutputStream 对象,并返回压缩后的临时文件。
希望这个回答可以帮助到您!
相关推荐
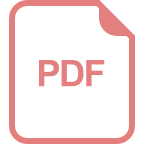
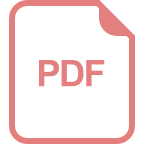
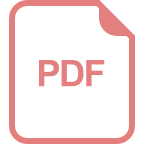












