代理服务器实现联网功能的java代码,可以用浏览器通过代理服务器上网,可通过dns服务器解析域名,可以识别http和url协议
时间: 2023-07-15 21:10:10 浏览: 37
以下是一个简单的代理服务器的Java代码示例,实现了通过代理服务器上网、DNS解析域名、识别HTTP和URL协议:
```java
import java.io.*;
import java.net.*;
public class ProxyServer {
private int port; // 代理服务器监听端口号
public ProxyServer(int port) {
this.port = port;
}
public void start() throws IOException {
ServerSocket serverSocket = new ServerSocket(port);
System.out.println("Proxy server started on port " + port);
while (true) {
Socket clientSocket = serverSocket.accept();
System.out.println("New client connected from " + clientSocket.getInetAddress());
// 为每个客户端连接创建一个新的线程处理请求
Thread requestThread = new Thread(() -> {
try {
// 从客户端读取请求
BufferedReader in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
String requestLine = in.readLine();
String[] requestParts = requestLine.split(" ");
String method = requestParts[0];
String url = requestParts[1];
// 解析目标主机和端口号
int port = 80; // 默认端口号为80
String host = null;
if (url.startsWith("http://")) {
host = url.substring(7);
int slashIndex = host.indexOf('/');
if (slashIndex != -1) {
host = host.substring(0, slashIndex);
}
} else {
host = url;
}
int colonIndex = host.indexOf(':');
if (colonIndex != -1) {
try {
port = Integer.parseInt(host.substring(colonIndex + 1));
} catch (NumberFormatException e) {
// 忽略端口号解析错误
}
host = host.substring(0, colonIndex);
}
// 解析HTTP头部,将其转发给目标服务器
String line;
StringBuilder headers = new StringBuilder();
while ((line = in.readLine()) != null && line.length() > 0) {
headers.append(line).append("\r\n");
}
headers.append("\r\n");
// 连接目标服务器
Socket serverSocket = new Socket(host, port);
OutputStream serverOut = serverSocket.getOutputStream();
// 将HTTP头部转发给目标服务器
serverOut.write(headers.toString().getBytes());
// 将客户端发送的请求体转发给目标服务器
if (method.equals("POST")) {
int contentLength = 0;
while ((line = in.readLine()) != null && line.length() > 0) {
if (line.startsWith("Content-Length:")) {
try {
contentLength = Integer.parseInt(line.substring(15).trim());
} catch (NumberFormatException e) {
// 忽略Content-Length解析错误
}
}
serverOut.write(line.getBytes());
serverOut.write("\r\n".getBytes());
}
serverOut.write("\r\n".getBytes());
if (contentLength > 0) {
char[] buffer = new char[contentLength];
in.read(buffer);
serverOut.write(new String(buffer).getBytes());
}
}
// 从目标服务器读取响应,并转发给客户端
InputStream serverIn = serverSocket.getInputStream();
OutputStream clientOut = clientSocket.getOutputStream();
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = serverIn.read(buffer)) != -1) {
clientOut.write(buffer, 0, bytesRead);
}
// 关闭连接
serverSocket.close();
clientSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
});
requestThread.start();
}
}
public static void main(String[] args) throws IOException {
ProxyServer proxyServer = new ProxyServer(8080);
proxyServer.start();
}
}
```
要使用这个代理服务器,只需在浏览器中设置HTTP代理为`localhost:8080`即可。注意,这个代理服务器只实现了最基本的功能,还有很多细节需要处理,例如HTTPS协议、缓存、安全认证等等。
相关推荐
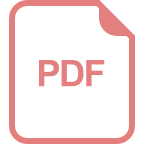
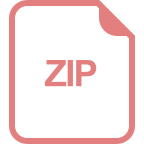
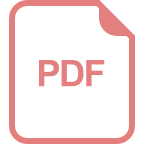














