用python编写程序,要求键盘输入一串字符,输出其中出现最多的字符以及该字符出现的次数。
时间: 2023-12-06 10:44:36 浏览: 166
可以通过Python中的字典来实现计算每个字符出现的次数,然后找出出现次数最多的字符。
以下是实现代码:
```python
string = input("请输入一串字符:")
char_dict = {}
# 遍历字符串中的每个字符,并计算每个字符出现的次数
for char in string:
if char in char_dict:
char_dict[char] += 1
else:
char_dict[char] = 1
# 找出出现次数最多的字符
max_count = 0
max_char = ''
for char, count in char_dict.items():
if count > max_count:
max_count = count
max_char = char
print("出现次数最多的字符是 %s,出现了 %d 次。" % (max_char, max_count))
```
运行代码后,输入一个字符串,程序会输出其中出现次数最多的字符以及该字符出现的次数。
相关问题
编写一个python程序,从键盘输入一个由小写字母组成的字符串,输出由出现次数最多的字母按升序组成的字符串
这个问题可以回答。以下是Python程序的代码:
```python
s = input("请输入一个由小写字母组成的字符串:")
count_dict = {}
for c in s:
if c in count_dict:
count_dict[c] += 1
else:
count_dict[c] = 1
max_count = max(count_dict.values())
result = "".join(sorted(c for c in count_dict if count_dict[c] == max_count))
print("出现次数最多的字母按升序组成的字符串是:", result)
```
假设输入的字符串是"hello world",程序的输出将是"lo",因为它们都出现了3次,而且按升序排列。
用python编写程序,实现从键盘输入一个字符串,去重后按字符升序输出
可以使用以下代码实现:
```python
string = input("请输入一个字符串:")
unique_chars = list(set(string))
unique_chars.sort()
print("去重后按字符升序输出:", "".join(unique_chars))
```
这段代码首先使用 `input()` 函数从键盘获取一个字符串,然后使用 `set()` 函数将字符串中的字符去重,再将去重后的字符转换为列表并使用 `sort()` 方法按升序排序。最后使用 `join()` 方法将排序后的字符列表转换为字符串并输出。
相关推荐
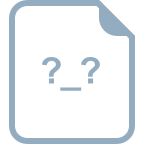












