taro返回上一级并刷新
时间: 2023-07-13 13:35:15 浏览: 356
您可以使用 `Taro.navigateBack` 方法返回上一级页面,并在上一级页面的 `onShow` 生命周期函数中重新加载数据。具体实现代码如下:
在需要刷新的页面中调用:
```javascript
// 在需要返回上一级页面并刷新的页面中调用
Taro.navigateBack({
delta: 1 // 返回上一级页面
})
```
在上一级页面的 `onShow` 生命周期函数中重新加载数据:
```javascript
// 在上一级页面的 onShow 生命周期函数中重新加载数据
onShow() {
// 加载数据的代码
}
```
这样就可以实现返回上一级并刷新的效果。
相关问题
taro中函数组件中点击Taro.navigateBack()返回上一页,点击时如何刷新上一页
在 Taro 中,从上一页返回时,上一页的 `componentWillReceiveProps` 生命周期函数不会被触发,因此默认情况下是不会刷新上一页的。但是你可以在上一页的 `componentDidShow` 生命周期函数中添加一些逻辑来实现返回时刷新的效果。
具体实现如下:
1. 在需要刷新的页面的 `componentDidShow` 函数中添加一个标记变量 `needRefresh`,并在组件 state 中定义该变量。
```jsx
class MyPage extends Component {
state = {
needRefresh: false
}
componentDidShow() {
if (this.state.needRefresh) {
// 执行刷新逻辑
this.setState({
needRefresh: false
})
}
}
// ...其他代码
}
```
2. 在需要返回的页面中使用 `Taro.navigateBack` 函数,并在 `success` 回调函数中将标记变量 `needRefresh` 置为 `true`。
```jsx
class NextPage extends Component {
handleClickBack() {
Taro.navigateBack({
success: () => {
const pages = Taro.getCurrentPages()
const prevPage = pages[pages.length - 2]
prevPage.setState({
needRefresh: true
})
}
})
}
// ...其他代码
}
```
这样,在返回到上一页时,就会触发 `componentDidShow` 函数,从而实现返回时刷新的效果。
taro 开发小程序实现下拉刷新功能
要在 Taro 中实现下拉刷新功能,你需要使用 Taro 提供的 `ScrollView` 组件,并在其中使用 `onScrollToLower` 属性来监听到达底部事件。以下是一个简单的示例代码:
```jsx
import { ScrollView, View } from '@tarojs/components';
import Taro from '@tarojs/taro';
class MyPage extends Taro.Component {
constructor(props) {
super(props);
this.state = {
dataList: [],
page: 1,
size: 10,
};
}
componentDidMount() {
// 页面加载时,执行一次下拉刷新
this.onPullDownRefresh();
}
async onPullDownRefresh() {
// 模拟异步加载数据
const newData = await this.loadData(this.state.page, this.state.size);
this.setState({
dataList: newData,
page: 1,
});
Taro.stopPullDownRefresh();
}
async onReachBottom() {
// 模拟异步加载数据
const newData = await this.loadData(this.state.page + 1, this.state.size);
this.setState({
dataList: [...this.state.dataList, ...newData],
page: this.state.page + 1,
});
}
async loadData(page, size) {
// 模拟异步加载数据
return new Promise((resolve) => {
setTimeout(() => {
const newData = Array.from({ length: size }, (_, i) => ({ id: (page - 1) * size + i, text: `数据${(page - 1) * size + i}` }));
resolve(newData);
}, 1000);
});
}
render() {
return (
<ScrollView scrollY style={{ height: '100vh' }} onScrollToLower={this.onReachBottom}>
<View>
{this.state.dataList.map((data) => (
<View key={data.id}>{data.text}</View>
))}
</View>
</ScrollView>
);
}
}
```
在上面的代码中,我们通过 `ScrollView` 组件来实现滚动,并通过 `onScrollToLower` 属性来监听到达底部事件,然后在事件回调函数中加载更多数据并更新状态。同时,我们也重写了 `onPullDownRefresh` 方法,来实现下拉刷新功能。在该方法中,我们模拟了异步加载数据的过程,并在加载完成后通过 `setState` 方法来更新状态,最后调用 `Taro.stopPullDownRefresh()` 方法来停止下拉刷新动画。
阅读全文
相关推荐
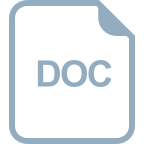
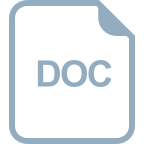
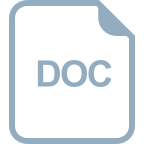
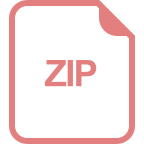












