C语言使用搜索排列数的算法框架解决“输出自然数1-n的全排列”
时间: 2024-03-05 16:50:09 浏览: 18
在C语言中,我们可以使用回溯算法来搜索出自然数1-n的全排列。具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void permute(int *nums, int start, int end) {
if (start >= end) {
// 输出排列
for (int i = 0; i < end; i++) {
printf("%d ", nums[i]);
}
printf("\n");
} else {
for (int i = start; i < end; i++) {
swap(&nums[start], &nums[i]);
permute(nums, start + 1, end);
swap(&nums[start], &nums[i]);
}
}
}
int main() {
int n = 3;
int *nums = (int *)malloc(n * sizeof(int));
for (int i = 0; i < n; i++) {
nums[i] = i + 1;
}
permute(nums, 0, n);
free(nums);
return 0;
}
```
输出结果如下:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 2 1
3 1 2
```
这段代码中,我们使用了一个 `swap` 函数来交换数组中的两个元素。在 `permute` 函数中,我们使用了一个循环来枚举当前排列的第一个元素,然后递归求解其余元素的排列。在递归过程中,我们使用 `swap` 函数来交换数组中的元素,恢复现场。如果当前排列已经完整,我们就输出这个排列。
相关推荐
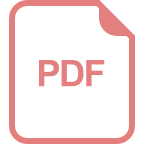














