Android studio 备忘录app完整的程序
时间: 2024-02-15 21:01:19 浏览: 147
抱歉,我无法提供完整的程序。但是,我可以提供一些基本的步骤和代码片段,以帮助你开始编写备忘录应用程序。
步骤:
1. 创建一个新的 Android Studio 项目。
2. 添加一个 RecyclerView 和一个 FloatingActionButton 来显示备忘录列表和添加备忘录按钮。
3. 创建一个备忘录数据模型类,包含标题、内容和日期等属性。
4. 创建一个备忘录适配器类,用于将备忘录数据绑定到 RecyclerView 上。
5. 创建一个数据库帮助类,用于创建和管理备忘录数据表。
6. 创建一个备忘录操作类,用于执行数据库操作,如添加、删除和更新备忘录。
7. 在 MainActivity 中初始化 RecyclerView、FloatingActionButton 和备忘录操作类。
8. 在 FloatingActionButton 的点击事件中启动一个新的 Activity,用于添加新的备忘录。
9. 在添加备忘录的 Activity 中,将用户输入的数据保存到数据库中。
10. 在 MainActivity 中,通过备忘录操作类查询数据库中的备忘录数据,并将其显示在 RecyclerView 上。
11. 在 RecyclerView 的每个备忘录项中,添加一个点击事件处理程序,以便用户可以查看和编辑备忘录。
12. 在查看和编辑备忘录的 Activity 中,将备忘录数据从数据库中加载并显示在相应的输入控件上。用户可以对备忘录进行编辑,然后将更改保存到数据库中。
代码片段:
备忘录数据模型类:
```
public class Memo {
private long id;
private String title;
private String content;
private String date;
public Memo(long id, String title, String content, String date) {
this.id = id;
this.title = title;
this.content = content;
this.date = date;
}
// getters and setters
}
```
备忘录适配器类:
```
public class MemoAdapter extends RecyclerView.Adapter<MemoAdapter.ViewHolder> {
private List<Memo> memoList;
public MemoAdapter(List<Memo> memoList) {
this.memoList = memoList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.memo_item, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
Memo memo = memoList.get(position);
holder.titleTextView.setText(memo.getTitle());
holder.contentTextView.setText(memo.getContent());
holder.dateTextView.setText(memo.getDate());
}
@Override
public int getItemCount() {
return memoList.size();
}
public static class ViewHolder extends RecyclerView.ViewHolder {
public TextView titleTextView;
public TextView contentTextView;
public TextView dateTextView;
public ViewHolder(View itemView) {
super(itemView);
titleTextView = itemView.findViewById(R.id.memo_title);
contentTextView = itemView.findViewById(R.id.memo_content);
dateTextView = itemView.findViewById(R.id.memo_date);
}
}
}
```
数据库帮助类:
```
public class MemoDbHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "memo.db";
private static final int DATABASE_VERSION = 1;
private static final String SQL_CREATE_MEMO_TABLE =
"CREATE TABLE " + MemoContract.MemoEntry.TABLE_NAME + " (" +
MemoContract.MemoEntry._ID + " INTEGER PRIMARY KEY AUTOINCREMENT," +
MemoContract.MemoEntry.COLUMN_TITLE + " TEXT NOT NULL," +
MemoContract.MemoEntry.COLUMN_CONTENT + " TEXT," +
MemoContract.MemoEntry.COLUMN_DATE + " TEXT NOT NULL)";
public MemoDbHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(SQL_CREATE_MEMO_TABLE);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
// not implemented yet
}
}
```
备忘录操作类:
```
public class MemoDao {
private SQLiteDatabase db;
public MemoDao(Context context) {
MemoDbHelper dbHelper = new MemoDbHelper(context);
db = dbHelper.getWritableDatabase();
}
public List<Memo> getAllMemos() {
List<Memo> memoList = new ArrayList<>();
Cursor cursor = db.query(
MemoContract.MemoEntry.TABLE_NAME,
null,
null,
null,
null,
null,
MemoContract.MemoEntry.COLUMN_DATE + " DESC");
while (cursor.moveToNext()) {
long id = cursor.getLong(cursor.getColumnIndex(MemoContract.MemoEntry._ID));
String title = cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_TITLE));
String content = cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_CONTENT));
String date = cursor.getString(cursor.getColumnIndex(MemoContract.MemoEntry.COLUMN_DATE));
Memo memo = new Memo(id, title, content, date);
memoList.add(memo);
}
cursor.close();
return memoList;
}
public void addMemo(Memo memo) {
ContentValues values = new ContentValues();
values.put(MemoContract.MemoEntry.COLUMN_TITLE, memo.getTitle());
values.put(MemoContract.MemoEntry.COLUMN_CONTENT, memo.getContent());
values.put(MemoContract.MemoEntry.COLUMN_DATE, memo.getDate());
db.insert(MemoContract.MemoEntry.TABLE_NAME, null, values);
}
public void updateMemo(Memo memo) {
ContentValues values = new ContentValues();
values.put(MemoContract.MemoEntry.COLUMN_TITLE, memo.getTitle());
values.put(MemoContract.MemoEntry.COLUMN_CONTENT, memo.getContent());
values.put(MemoContract.MemoEntry.COLUMN_DATE, memo.getDate());
String selection = MemoContract.MemoEntry._ID + " = ?";
String[] selectionArgs = { String.valueOf(memo.getId()) };
db.update(MemoContract.MemoEntry.TABLE_NAME, values, selection, selectionArgs);
}
public void deleteMemo(long id) {
String selection = MemoContract.MemoEntry._ID + " = ?";
String[] selectionArgs = { String.valueOf(id) };
db.delete(MemoContract.MemoEntry.TABLE_NAME, selection, selectionArgs);
}
}
```
阅读全文
相关推荐
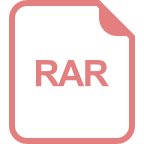
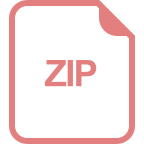
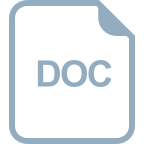





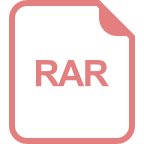
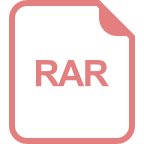
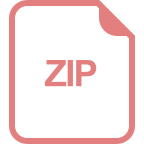
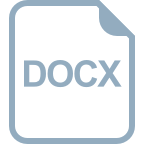
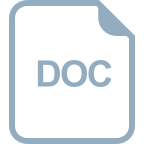
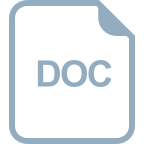



