帮我生成上述AA-ICP点云配准的代码
时间: 2024-01-12 09:05:39 浏览: 28
好的,以下是一个简单的AA-ICP点云配准的Python代码示例:
```python
import numpy as np
from scipy.spatial import KDTree
def aa_icp(source, target, max_iterations=50, tolerance=1e-5):
"""
:param source: numpy array, shape (N, 3), source point cloud
:param target: numpy array, shape (M, 3), target point cloud
:param max_iterations: int, maximum number of iterations
:param tolerance: float, convergence tolerance
:return R: numpy array, shape (3, 3), rotation matrix
:return t: numpy array, shape (3,), translation vector
"""
# Initialize transformation
R = np.eye(3)
t = np.zeros(3)
# Build KDTree for target points
tree = KDTree(target)
# Iterate until convergence
for i in range(max_iterations):
# Find nearest neighbors
distances, indices = tree.query(source)
# Compute centroid of source and target points
centroid_source = np.mean(source, axis=0)
centroid_target = np.mean(target[indices], axis=0)
# Compute centered source and target points
centered_source = source - centroid_source
centered_target = target[indices] - centroid_target
# Compute covariance matrix
H = np.dot(centered_target.T, centered_source)
# Compute SVD of covariance matrix
U, _, Vt = np.linalg.svd(H)
# Compute rotation matrix
R = np.dot(Vt.T, U.T)
# Compute translation vector
t = centroid_target - np.dot(R, centroid_source)
# Transform source points
transformed_source = np.dot(R, source.T).T + t
# Compute error
error = np.mean(np.linalg.norm(transformed_source - target[indices], axis=1))
# Check convergence
if error < tolerance:
break
# Update source points for next iteration
source = transformed_source
return R, t
```
这段代码实现了AA-ICP点云配准的主要步骤,包括建立KDTree,计算中心点,计算协方差矩阵,通过奇异值分解计算旋转矩阵和平移向量,以及更新源点云等。您可以将自己的点云数据传递给这个函数,并得到旋转矩阵和平移向量。
相关推荐
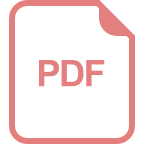














