请帮忙用QT写一个蓝牙app检测系统
时间: 2024-06-10 17:08:02 浏览: 112
好的,关于您的问题,我可以为您提供一些帮助。您可以使用QT开发环境,结合蓝牙模块进行开发。首先,您需要了解蓝牙模块的相关知识,包括蓝牙协议、蓝牙设备的连接方式和基本操作等。然后,您可以利用QT提供的相关API、库来实现蓝牙设备的搜索、连接、发送和接收信息等功能。最后,您需要进行测试和调试,确保软件的稳定性和可靠性。希望这些信息可以对您有所帮助。
相关问题
请帮忙用QT写一个蓝牙app检测系统程序代码
好的,我可以回答这个问题。以下是使用 Qt 编写蓝牙检测程序的示例代码:
```cpp
#include <QCoreApplication>
#include <QBluetoothDeviceDiscoveryAgent>
#include <QBluetoothDeviceInfo>
#include <QDebug>
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
// 创建蓝牙设备发现代理
QBluetoothDeviceDiscoveryAgent agent;
// 当发现新设备时,打印出设备名称和 MAC 地址
QObject::connect(&agent, &QBluetoothDeviceDiscoveryAgent::deviceDiscovered, [&](const QBluetoothDeviceInfo &device) {
qDebug() << "Discovered new device:" << device.name() << device.address().toString();
});
// 开始搜索蓝牙设备
agent.start();
// 运行程序事件循环
return QCoreApplication::exec();
}
```
这个程序会搜索附近的蓝牙设备,并在控制台输出它们的名称和 MAC 地址。你可以根据需要修改这个程序来实现你想要的蓝牙检测功能。
用QT写一个蓝牙app
好的,下面是一个简单的蓝牙app示例:
首先,在Qt Creator中创建一个新的Qt Widgets Application项目,并在项目中添加以下头文件:
```c++
#include <QWidget>
#include <QBluetoothDeviceDiscoveryAgent>
#include <QBluetoothLocalDevice>
#include <QBluetoothSocket>
```
然后,在主窗口的头文件中定义以下私有变量:
```c++
private:
QBluetoothDeviceDiscoveryAgent *m_discoveryAgent; // 蓝牙设备发现代理
QBluetoothLocalDevice *m_localDevice; // 本地蓝牙设备
QBluetoothSocket *m_socket; // 蓝牙socket
```
在主窗口的构造函数中初始化这些变量:
```c++
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
// 初始化蓝牙设备发现代理
m_discoveryAgent = new QBluetoothDeviceDiscoveryAgent(this);
connect(m_discoveryAgent, SIGNAL(deviceDiscovered(QBluetoothDeviceInfo)), this, SLOT(deviceDiscovered(QBluetoothDeviceInfo)));
// 初始化本地蓝牙设备
m_localDevice = new QBluetoothLocalDevice(this);
// 初始化蓝牙socket
m_socket = new QBluetoothSocket(QBluetoothServiceInfo::RfcommProtocol, this);
}
```
接下来,实现以下槽函数:
```c++
// 开始扫描蓝牙设备
void MainWindow::on_scanButton_clicked()
{
ui->deviceListWidget->clear(); // 清空设备列表
m_discoveryAgent->start(); // 开始扫描
}
// 停止扫描蓝牙设备
void MainWindow::on_stopButton_clicked()
{
m_discoveryAgent->stop(); // 停止扫描
}
// 发现蓝牙设备
void MainWindow::deviceDiscovered(const QBluetoothDeviceInfo &device)
{
ui->deviceListWidget->addItem(device.name() + " (" + device.address().toString() + ")"); // 将设备添加到列表中
}
// 连接到选定的蓝牙设备
void MainWindow::on_connectButton_clicked()
{
QString deviceName = ui->deviceListWidget->currentItem()->text(); // 获取选定的设备名称
deviceName = deviceName.split("(").first().trimmed(); // 提取设备名称
QBluetoothDeviceInfo deviceInfo; // 创建设备信息对象
foreach (const QBluetoothDeviceInfo &info, m_discoveryAgent->discoveredDevices()) {
if (info.name() == deviceName) {
deviceInfo = info;
break;
}
}
if (!deviceInfo.isValid()) { // 如果设备信息无效,则返回
return;
}
m_socket->connectToService(deviceInfo.address(), QBluetoothUuid(QBluetoothUuid::SerialPort)); // 连接蓝牙设备
}
// 断开蓝牙设备连接
void MainWindow::on_disconnectButton_clicked()
{
m_socket->disconnectFromService(); // 断开连接
}
```
最后,在主窗口的析构函数中释放这些变量:
```c++
MainWindow::~MainWindow()
{
delete m_discoveryAgent;
delete m_localDevice;
delete m_socket;
}
```
这样,一个简单的蓝牙app就完成了。你可以在UI中添加更多的按钮和控件来实现更多的功能。
阅读全文
相关推荐
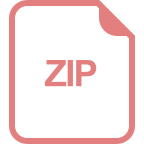
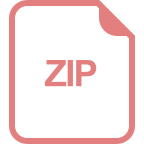













