已知图的邻接矩阵,请用C语言编写函数对图进行深度遍历和广度遍历
时间: 2024-05-15 22:14:44 浏览: 14
好的,我理解了您的要求。以下是对图进行深度遍历和广度遍历的 C 语言代码示例:
```C
#include <stdio.h>
#include <stdlib.h>
// 定义邻接矩阵类型
#define MAX_SIZE 100
typedef struct {
int vertex[MAX_SIZE];
int edge[MAX_SIZE][MAX_SIZE];
int vertex_num, edge_num;
} MGraph;
// 定义队列类型和相关操作
#define QUEUE_SIZE 100
typedef struct {
int data[QUEUE_SIZE];
int front, rear;
} Queue;
void init_queue(Queue *q) {
q->front = q->rear = 0;
}
int is_queue_empty(Queue *q) {
return q->front == q->rear;
}
int enqueue(Queue *q, int x) {
if ((q->rear + 1) % QUEUE_SIZE == q->front) {
return 0; // 队列已满
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % QUEUE_SIZE;
return 1; // 入队成功
}
int dequeue(Queue *q, int *x) {
if (is_queue_empty(q)) {
return 0; // 队列已空
}
*x = q->data[q->front];
q->front = (q->front + 1) % QUEUE_SIZE;
return 1; // 出队成功
}
// 深度优先遍历
void dfs(int v, int visited[], MGraph *graph) {
int i;
printf("%d ", graph->vertex[v]);
visited[v] = 1;
for (i = 0; i < graph->vertex_num; i++) {
if (graph->edge[v][i] && !visited[i]) {
dfs(i, visited, graph);
}
}
}
void dfs_traverse(MGraph *graph) {
int visited[MAX_SIZE] = {0};
int i;
for (i = 0; i < graph->vertex_num; i++) {
if (!visited[i]) {
dfs(i, visited, graph);
}
}
}
// 广度优先遍历
void bfs_traverse(MGraph *graph) {
int visited[MAX_SIZE] = {0};
Queue q;
int i, v;
for (i = 0; i < graph->vertex_num; i++) {
if (!visited[i]) {
visited[i] = 1;
printf("%d ", graph->vertex[i]);
enqueue(&q, i);
while (!is_queue_empty(&q)) {
dequeue(&q, &v);
for (i = 0; i < graph->vertex_num; i++) {
if (graph->edge[v][i] && !visited[i]) {
visited[i] = 1;
printf("%d ", graph->vertex[i]);
enqueue(&q, i);
}
}
}
}
}
}
// 测试函数
int main() {
MGraph graph = {{
1, 2, 3, 4, 5, 6
}, {
{0, 1, 1, 0, 0, 0},
{1, 0, 0, 1, 0, 0},
{1, 0, 0, 1, 1, 0},
{0, 1, 1, 0, 0, 1},
{0, 0, 1, 0, 0, 1},
{0, 0, 0, 1, 1, 0}
}, 6, 9};
printf("深度优先遍历:\n");
dfs_traverse(&graph);
printf("\n广度优先遍历:\n");
bfs_traverse(&graph);
return 0;
}
```
这份代码演示了两种遍历方式的实现:深度优先遍历和广度优先遍历。其中,深度优先遍历使用递归方式实现,广度优先遍历使用队列实现。在测试函数中,我们使用了一个包含 6 个节点和 9 条边的简单图作为数据输入。您可以根据需要修改处于注释中的代码来适应不同的图数据输入。
相关推荐
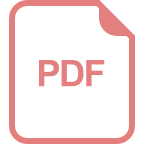
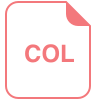
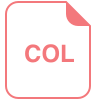
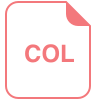
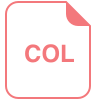
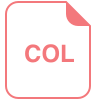









