哈夫曼编码c语言源代码
时间: 2024-10-19 07:15:43 浏览: 72
哈夫曼编码是一种基于字符频率的压缩算法,常用于数据存储和传输的优化。在C语言中实现哈夫曼编码,你需要创建一个优先队列、构建哈夫曼树并生成编码。下面是一个简单的示例,展示了如何实现哈夫曼编码的基本步骤:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <queue>
typedef struct Node {
char data;
int freq;
struct Node *left, *right;
} Node;
Node* newNode(char data, int freq) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->freq = freq;
node->left = node->right = NULL;
return node;
}
bool compare(const void* a, const void* b) {
return ((Node*)a)->freq > ((Node*)b)->freq;
}
Node* buildHuffmanTree(Node** nodes, int count) {
if (count <= 1)
return nodes[0];
priority_queue<Node*, vector<Node*>, decltype(compare)> pq(compare);
for (int i = 0; i < count; i++)
pq.push(nodes[i]);
while (pq.size() > 1) {
Node* left = pq.top(); pq.pop();
Node* right = pq.top(); pq.pop();
// Merge two nodes into a new one with their sum frequency
Node* merged = newNode('\0', left->freq + right->freq);
merged->left = left;
merged->right = right;
pq.push(merged);
}
return pq.top();
}
void printCodes(Node* root, char* codes, int index, char code) {
if (!root->left && !root->right) {
codes[root->data] = code;
printf("%c -> %d\n", root->data, index++);
} else {
printCodes(root->left, codes, index, '0');
printCodes(root->right, codes, index, '1');
}
}
int main() {
// ... 具体字符及其频率的输入或初始化 ...
Node** nodes = malloc(sizeof(Node*) * count);
// ... 将节点添加到数组中 ...
Node* huffTree = buildHuffmanTree(nodes, count);
char* codes = (char*)malloc(count * sizeof(char));
memset(codes, '\0', count);
printCodes(huffTree, codes, 0, '0');
free(nodes);
free(huffTree);
return 0;
}
```
这个示例包括了创建节点、构造哈夫曼树以及打印编码的过程。实际应用中,你需要处理用户输入的字符频率,并将生成的哈夫曼树用于编码数据。
阅读全文
相关推荐
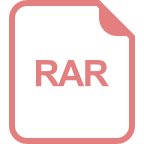
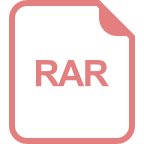
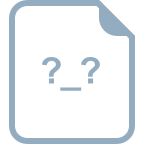
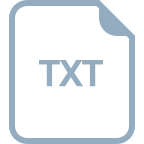
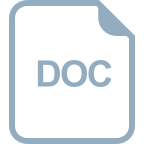
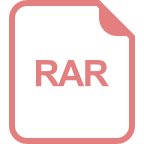
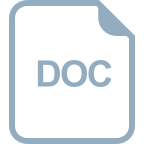
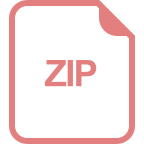
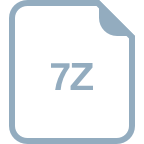
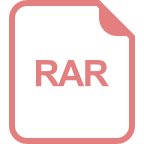
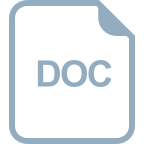
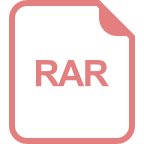
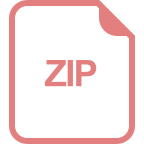
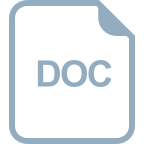
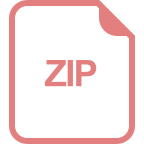
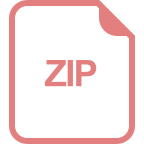
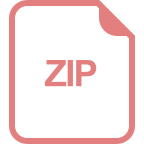
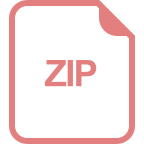