用C语言写一个判断倍数的函数
时间: 2024-01-21 21:16:32 浏览: 34
以下是用C语言编写的判断倍数的函数:
```c
#include <stdio.h>
// 判断num是否是factor的倍数
int is_multiple(int num, int factor) {
if (num % factor == 0) {
return 1; // 是倍数,返回1
} else {
return 0; // 不是倍数,返回0
}
}
int main() {
int num, factor;
printf("请输入一个整数num:");
scanf("%d", &num);
printf("请输入一个整数factor:");
scanf("%d", &factor);
if (is_multiple(num, factor)) {
printf("%d是%d的倍数\n", num, factor);
} else {
printf("%d不是%d的倍数\n", num, factor);
}
return 0;
}
```
运行程序,输入一个整数和一个因子,程序会输出判断结果。例如输入num为10,factor为5,程序会输出“10是5的倍数”。
相关问题
用C语言写一个最小公倍数的函数
```
#include <stdio.h>
int lcm(int a, int b);
int main()
{
int num1, num2, LCM;
printf("输入两个正整数:");
scanf("%d %d", &num1, &num2);
if (num1 <= 0 || num2 <= 0)
{
printf("输入的数字必须是正整数\n");
return 0;
}
LCM = lcm(num1, num2);
printf("最小公倍数为:%d\n", LCM);
return 0;
}
int lcm(int a, int b)
{
int max;
max = (a > b) ? a : b;
while (1)
{
if (max % a == 0 && max % b == 0)
return max;
++max;
}
}
```
这个函数接收两个正整数作为参数,并返回它们的最小公倍数。它首先检查输入数据是否是正整数,然后使用一个循环来计算最小公倍数。
用C语言写一个灰度图像放大函数
好的,下面是一个简单的用C语言实现灰度图像放大的函数:
```
void zoom_in(unsigned char* img, int width, int height, int zoom_factor) {
int new_width = width * zoom_factor;
int new_height = height * zoom_factor;
unsigned char* new_img = (unsigned char*)malloc(new_width * new_height * sizeof(unsigned char));
for (int i = 0; i < new_height; i++) {
for (int j = 0; j < new_width; j++) {
int x = j / zoom_factor;
int y = i / zoom_factor;
int index = i * new_width + j;
int old_index = y * width + x;
new_img[index] = img[old_index];
}
}
memcpy(img, new_img, new_width * new_height * sizeof(unsigned char));
free(new_img);
}
```
该函数会接受一个指向灰度图像数据的指针,图像的宽度和高度,以及要放大的倍数。它会创建一个新的数组来存储放大后的图像,并将原始图像中的像素插入到适当的位置。最后,它会用放大后的图像替换原始图像。
注意,这个函数只能用于灰度图像,如果要处理彩色图像,需要对每个通道分别进行处理。此外,这个函数可能会引起内存溢出,因此在实际使用时需要注意内存管理。
相关推荐
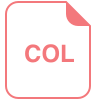
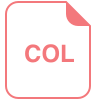
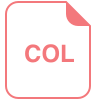









