已知截止频率Pf,Q,增益Gain,在QT中使用C++绘制频响曲线lowshelf和highshelf
时间: 2024-02-17 08:03:02 浏览: 124
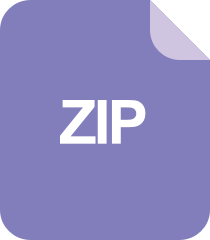
基于QT的C++编程
好的,我理解了你的问题。在QT中可以使用QCustomPlot库来实现绘制频响曲线的功能。下面是示例代码:
```c++
#include "qcustomplot.h"
void drawLowShelf(QCustomPlot *customPlot, double Pf, double Q, double Gain)
{
customPlot->addGraph(); // 添加一条曲线
double w0 = 2 * M_PI * Pf;
double alpha = sin(w0) / (2 * Q);
double A = pow(10, Gain / 40);
double sqrtA = sqrt(A);
QVector<double> x(1001), y(1001); // 定义横坐标和纵坐标的向量
for (int i = 0; i <= 1000; ++i)
{
double w = i / 1000.0 * M_PI;
double cosw = cos(w);
double sinw = sin(w);
double b0 = A * ((A + 1) - (A - 1) * cosw + 2 * sqrtA * alpha * sinw);
double b1 = 2 * A * ((A - 1) - (A + 1) * cosw);
double b2 = A * ((A + 1) - (A - 1) * cosw - 2 * sqrtA * alpha * sinw);
double a0 = (A + 1) + (A - 1) * cosw + 2 * sqrtA * alpha * sinw;
double a1 = -2 * ((A - 1) + (A + 1) * cosw);
double a2 = (A + 1) + (A - 1) * cosw - 2 * sqrtA * alpha * sinw;
double H = (b0 - b1 + b2) / a0;
x[i] = w / M_PI;
y[i] = H;
}
customPlot->graph(0)->setData(x, y); // 设置曲线的数据
customPlot->rescaleAxes(); // 调整坐标轴范围
customPlot->replot(); // 重新绘制曲线
}
void drawHighShelf(QCustomPlot *customPlot, double Pf, double Q, double Gain)
{
customPlot->addGraph(); // 添加一条曲线
double w0 = 2 * M_PI * Pf;
double alpha = sin(w0) / (2 * Q);
double A = pow(10, Gain / 40);
double sqrtA = sqrt(A);
QVector<double> x(1001), y(1001); // 定义横坐标和纵坐标的向量
for (int i = 0; i <= 1000; ++i)
{
double w = i / 1000.0 * M_PI;
double cosw = cos(w);
double sinw = sin(w);
double b0 = A * ((A + 1) + (A - 1) * cosw + 2 * sqrtA * alpha * sinw);
double b1 = -2 * A * ((A - 1) + (A + 1) * cosw);
double b2 = A * ((A + 1) + (A - 1) * cosw - 2 * sqrtA * alpha * sinw);
double a0 = (A + 1) - (A - 1) * cosw + 2 * sqrtA * alpha * sinw;
double a1 = 2 * ((A - 1) - (A + 1) * cosw);
double a2 = (A + 1) - (A - 1) * cosw - 2 * sqrtA * alpha * sinw;
double H = (b0 - b1 + b2) / a0;
x[i] = w / M_PI;
y[i] = H;
}
customPlot->graph(0)->setData(x, y); // 设置曲线的数据
customPlot->rescaleAxes(); // 调整坐标轴范围
customPlot->replot(); // 重新绘制曲线
}
```
这段代码中,我们分别定义了 `drawLowShelf` 和 `drawHighShelf` 函数来绘制低置信度和高置信度的频响曲线。这两个函数的参数分别是截止频率 `Pf`、品质因数 `Q` 和增益 `Gain`,以及一个 `QCustomPlot` 指针,用于指向需要绘图的 `QCustomPlot` 对象。
在这两个函数中,我们首先计算出一些必要的系数,然后对于每一个横坐标值(从 0 到 1),都计算出相应的纵坐标值,并将它们存储在 `x` 和 `y` 向量中。最后,我们使用 `setData` 函数将这些数据设置给 `QCustomPlot` 对象,然后使用 `rescaleAxes` 和 `replot` 函数调整坐标轴范围并重新绘制曲线。
使用这两个函数来绘制频响曲线的示例代码如下:
```c++
#include <QApplication>
#include <QMainWindow>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QMainWindow window;
window.resize(800, 600);
QCustomPlot *customPlot = new QCustomPlot(&window);
customPlot->setGeometry(0, 0, 800, 600);
customPlot->xAxis->setLabel("Frequency (Hz)");
customPlot->yAxis->setLabel("Gain (dB)");
drawLowShelf(customPlot, 1000, 0.7, 3);
drawHighShelf(customPlot, 5000, 0.5, -6);
window.show();
return app.exec();
}
```
这段代码中,我们创建了一个 `QMainWindow` 对象,并在其中添加了一个 `QCustomPlot` 对象。我们将这个 `QCustomPlot` 对象的坐标轴标签设置为 "Frequency (Hz)" 和 "Gain (dB)",然后分别调用 `drawLowShelf` 和 `drawHighShelf` 函数来绘制低置信度和高置信度的频响曲线。
最后,我们将这个 `QMainWindow` 对象显示出来,并启动 Qt 应用程序。运行程序后,你应该可以看到绘制出的频响曲线。
阅读全文
相关推荐


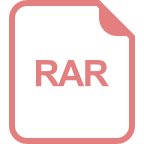
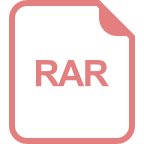
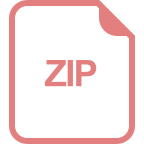
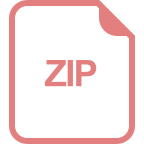
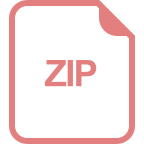
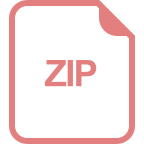
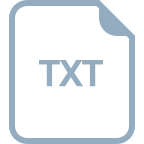
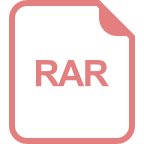
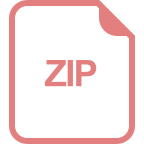
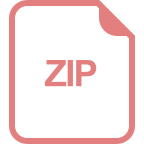
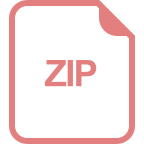
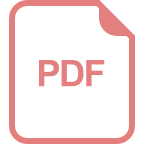