c++用ffmpeg创建rtsp服务器
时间: 2023-10-05 16:11:43 浏览: 198
要用C++创建RTSP服务器,需要使用FFmpeg库。下面是一个简单的示例代码:
```c++
#include <iostream>
#include <cstdio>
#include <cstdlib>
#include <cstring>
#include <sstream>
#include <thread>
#include <chrono>
extern "C" {
#include <libavutil/timestamp.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
}
#define RTSP_PORT 554
#define RTSP_TIMEOUT 2000000
int main(int argc, char* argv[]) {
av_register_all();
AVFormatContext* av_format_ctx = NULL;
AVOutputFormat* av_output_fmt = NULL;
AVStream* av_stream = NULL;
AVCodecContext* av_codec_ctx = NULL;
AVCodec* av_codec = NULL;
AVDictionary* av_opts = NULL;
std::ostringstream rtsp_url;
rtsp_url << "rtsp://localhost:" << RTSP_PORT << "/test";
std::ostringstream video_size;
video_size << "640x480";
std::ostringstream frame_rate;
frame_rate << "30";
avformat_alloc_output_context2(&av_format_ctx, NULL, "rtsp", rtsp_url.str().c_str());
if (!av_format_ctx) {
std::cerr << "Failed to allocate output context" << std::endl;
return -1;
}
av_output_fmt = av_format_ctx->oformat;
if (av_output_fmt->video_codec == AV_CODEC_ID_NONE) {
std::cerr << "Output format does not support video encoding" << std::endl;
return -1;
}
av_stream = avformat_new_stream(av_format_ctx, NULL);
if (!av_stream) {
std::cerr << "Failed to create new stream" << std::endl;
return -1;
}
av_codec_ctx = av_stream->codec;
av_codec_ctx->codec_id = av_output_fmt->video_codec;
av_codec_ctx->codec_type = AVMEDIA_TYPE_VIDEO;
av_codec_ctx->bit_rate = 400000;
av_codec_ctx->width = 640;
av_codec_ctx->height = 480;
av_codec_ctx->time_base = (AVRational){1, atoi(frame_rate.str().c_str())};
av_codec_ctx->gop_size = 10;
av_codec = avcodec_find_encoder(av_codec_ctx->codec_id);
if (!av_codec) {
std::cerr << "Failed to find encoder" << std::endl;
return -1;
}
if (avcodec_open2(av_codec_ctx, av_codec, &av_opts) < 0) {
std::cerr << "Failed to open codec" << std::endl;
return -1;
}
av_dict_free(&av_opts);
avio_open(&av_format_ctx->pb, rtsp_url.str().c_str(), AVIO_FLAG_WRITE);
int ret = avformat_write_header(av_format_ctx, &av_opts);
if (ret < 0) {
std::cerr << "Failed to write header" << std::endl;
return -1;
}
AVFrame* av_frame = av_frame_alloc();
av_frame->format = av_codec_ctx->pix_fmt;
av_frame->width = av_codec_ctx->width;
av_frame->height = av_codec_ctx->height;
av_frame->pts = 0;
avpicture_fill((AVPicture*)av_frame, NULL, av_codec_ctx->pix_fmt, av_codec_ctx->width, av_codec_ctx->height);
AVPacket av_packet;
av_init_packet(&av_packet);
av_packet.data = NULL;
av_packet.size = 0;
int frame_count = 0;
while (1) {
std::this_thread::sleep_for(std::chrono::milliseconds(33));
av_frame->pts = frame_count;
if (avcodec_send_frame(av_codec_ctx, av_frame) < 0) {
std::cerr << "Failed to send frame" << std::endl;
break;
}
while (avcodec_receive_packet(av_codec_ctx, &av_packet) == 0) {
av_packet.stream_index = av_stream->index;
av_packet.pts = av_rescale_q_rnd(av_packet.pts, av_codec_ctx->time_base, av_stream->time_base, AV_ROUND_NEAR_INF | AV_ROUND_PASS_MINMAX);
av_packet.dts = av_rescale_q_rnd(av_packet.dts, av_codec_ctx->time_base, av_stream->time_base, AV_ROUND_NEAR_INF | AV_ROUND_PASS_MINMAX);
av_packet.duration = av_rescale_q(av_packet.duration, av_codec_ctx->time_base, av_stream->time_base);
av_packet.pos = -1;
if (av_packet.pts < 0) {
av_packet.pts = 0;
}
if (av_packet.dts < 0) {
av_packet.dts = 0;
}
ret = av_interleaved_write_frame(av_format_ctx, &av_packet);
if (ret < 0) {
std::cerr << "Failed to write frame" << std::endl;
break;
}
av_packet_unref(&av_packet);
}
++frame_count;
}
av_write_trailer(av_format_ctx);
if (av_codec_ctx) {
avcodec_close(av_codec_ctx);
avcodec_free_context(&av_codec_ctx);
}
if (av_format_ctx) {
avio_closep(&av_format_ctx->pb);
avformat_free_context(av_format_ctx);
}
return 0;
}
```
这个代码使用了FFmpeg库来创建一个RTSP服务器,它生成一些假数据来模拟一个视频流。你需要确保已经安装了FFmpeg,并且已经将其链接到你的项目中。你可以使用cmake来构建你的项目,并将FFmpeg链接到它。
阅读全文
相关推荐
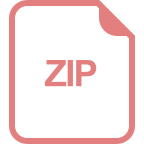
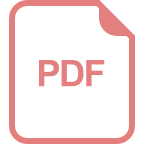






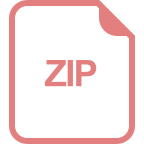