使用 C++ 和 FFmpeg 创建 RTSP 服务器
时间: 2024-06-05 17:11:24 浏览: 213
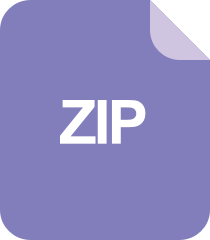
RTSP服务器 c++

要使用 C++ 和 FFmpeg 创建 RTSP 服务器,需要进行以下步骤:
1. 安装 FFmpeg 库:首先需要从 FFmpeg 的官网下载最新的 FFmpeg 库,并将其安装在本地环境中。
2. 创建 RTSP 服务器:使用 C++ 编写一个 RTSP 服务器程序,该程序将使用 FFmpeg 库来处理音视频流。可以使用开源的库,如 live555 或者 EasyDarwin,来创建 RTSP 服务器。
3. 加载音视频流:使用 FFmpeg 库加载音视频流,并将其编码为 RTSP 可以识别的格式。可以使用 FFmpeg 的示例代码来加载音视频流。
4. 发送音视频流:使用 FFmpeg 库将编码后的音视频流发送到 RTSP 服务器中。可以使用 FFmpeg 的示例代码来发送音视频流。
5. 启动 RTSP 服务器:将编写好的 C++ 代码编译成可执行文件,并在服务器上运行该文件,即可启动 RTSP 服务器。
需要注意的是,创建 RTSP 服务器需要一定的编程经验和 FFmpeg 库的基础知识。建议先熟悉 FFmpeg 的使用方法和 RTSP 协议的原理,再进行编程。
阅读全文
相关推荐
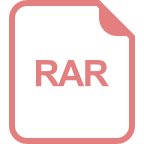







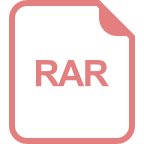
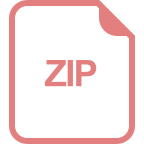
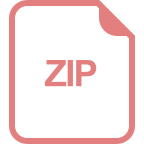