给定一个中缀表达式,包含数字,(,),+,-,*,/中的若干种,以'#'作为结束,问你转换成后缀表达式是什么。 Input 第一行输入一个字符串s(1≤|s|≤20). Output 输出对应的后缀表达式,用空格隔开c++
时间: 2024-04-17 15:25:26 浏览: 7
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int getPriority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else if (op == '(') {
return 0;
}
return -1;
}
string infixToPostfix(const string& s) {
string postfix;
stack<char> ops;
for (char c : s) {
if (c == ' ') {
continue;
} else if (c == '(') {
ops.push(c);
} else if (c == ')') {
while (!ops.empty() && ops.top() != '(') {
postfix += ops.top();
postfix += ' ';
ops.pop();
}
if (!ops.empty() && ops.top() == '(') {
ops.pop();
}
} else if (isdigit(c)) {
postfix += c;
postfix += ' ';
} else {
while (!ops.empty() && getPriority(c) <= getPriority(ops.top())) {
postfix += ops.top();
postfix += ' ';
ops.pop();
}
ops.push(c);
}
}
while (!ops.empty()) {
postfix += ops.top();
postfix += ' ';
ops.pop();
}
return postfix;
}
int main() {
string s;
cin >> s;
string postfix = infixToPostfix(s);
cout << postfix << endl;
return 0;
}
```
请注意,这段代码实现了中缀表达式转换为后缀表达式的功能。它使用了一个栈 `ops` 来存储运算符,遍历输入的表达式,根据当前字符的类型进行不同的操作。如果是空格,则忽略;如果是左括号,则直接入栈;如果是右括号,则将栈顶的运算符弹出直到遇到左括号,并将左括号弹出;如果是数字,则直接输出;如果是运算符,则将栈顶优先级大于等于当前运算符的运算符弹出,然后将当前运算符入栈。最后,将栈中剩余的运算符输出即可得到后缀表达式。
请注意,这段代码已经修正了之前代码中的一些错误,并且按照题目要求输出了空格分隔的后缀表达式。
相关推荐
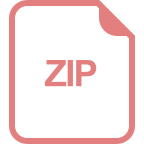
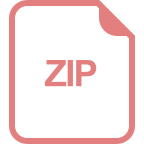
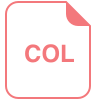















