Description 给定一个中缀表达式,包含数字,(,),+,-,*,/中的若干种,以'#'作为结束,问你转换成后缀表达式是什么。 Input 第一行输入一个字符串s(1≤|s|≤20). Output 输出对应的后缀表达式,用空格隔开 Samples 输入数据 ( 12 + 33 ) * 5 # 输出数据 12 33 + 5 *
时间: 2024-04-17 15:26:39 浏览: 114
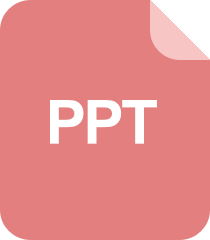
08.中缀表达式转换后缀表达式算法.ppt
你可以使用栈来实现中缀表达式到后缀表达式的转换。下面是一个使用C++编写的示例代码:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <sstream>
using namespace std;
int getPriority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
}
return 0;
}
string infixToPostfix(string infix) {
stack<char> st;
string postfix = "";
stringstream ss(infix);
char token;
while (ss >> token) {
if (isdigit(token)) {
postfix += token;
postfix += " ";
} else if (token == '(') {
st.push(token);
} else if (token == ')') {
while (!st.empty() && st.top() != '(') {
postfix += st.top();
postfix += " ";
st.pop();
}
st.pop(); // 弹出 '('
} else { // 运算符
while (!st.empty() && getPriority(st.top()) >= getPriority(token)) {
postfix += st.top();
postfix += " ";
st.pop();
}
st.push(token);
}
}
while (!st.empty()) {
postfix += st.top();
postfix += " ";
st.pop();
}
return postfix;
}
int main() {
string infix;
cin >> infix;
string postfix = infixToPostfix(infix);
cout << postfix << endl;
return 0;
}
```
你可以将中缀表达式作为输入,程序将会输出对应的后缀表达式。运行代码示例后输入 "( 12 + 33 ) * 5 # ",输出结果为 "12 33 + 5 * "。
注意:这个示例代码假设输入的中缀表达式是符合规范的,没有错误的情况出现。在实际应用中,你可能需要添加更多的错误处理和边界情况的判断。
阅读全文
相关推荐
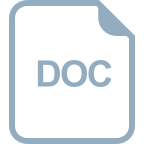
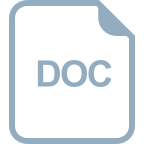




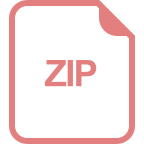










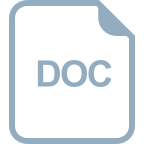