java完成自定义链表结构 1.添加数据 2.删除数据 3.修改数据 4.获得数据个数 5.通过索引获得数据 6.查询链表数据是否存在
时间: 2024-02-28 16:57:59 浏览: 90
好的,以下是一个简单的Java自定义链表结构实现,包含您提到的功能:
```java
public class MyLinkedList {
private Node head;
private int size;
// 添加数据
public void add(Object data) {
if (head == null) {
head = new Node(data);
} else {
Node node = head;
while (node.next != null) {
node = node.next;
}
node.next = new Node(data);
}
size++;
}
// 删除数据
public void remove(Object data) {
if (head == null) {
return;
}
if (head.data.equals(data)) {
head = head.next;
size--;
return;
}
Node prev = head;
Node curr = head.next;
while (curr != null) {
if (curr.data.equals(data)) {
prev.next = curr.next;
size--;
return;
}
prev = curr;
curr = curr.next;
}
}
// 修改数据
public boolean update(int index, Object data) {
if (index < 0 || index >= size) {
return false;
}
Node node = head;
for (int i = 0; i < index; i++) {
node = node.next;
}
node.data = data;
return true;
}
// 获得数据个数
public int size() {
return size;
}
// 通过索引获得数据
public Object get(int index) {
if (index < 0 || index >= size) {
return null;
}
Node node = head;
for (int i = 0; i < index; i++) {
node = node.next;
}
return node.data;
}
// 查询链表数据是否存在
public boolean contains(Object data) {
if (head == null) {
return false;
}
Node node = head;
while (node != null) {
if (node.data.equals(data)) {
return true;
}
node = node.next;
}
return false;
}
private class Node {
Object data;
Node next;
public Node(Object data) {
this.data = data;
}
}
}
```
使用方法:
```java
MyLinkedList list = new MyLinkedList();
list.add("A");
list.add("B");
list.add("C");
System.out.println(list.size()); // 3
list.remove("B");
System.out.println(list.size()); // 2
list.update(0, "D");
System.out.println(list.get(0)); // D
System.out.println(list.contains("C")); // true
System.out.println(list.contains("E")); // false
```
希望对您有所帮助!
阅读全文
相关推荐
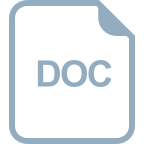
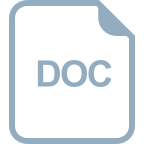
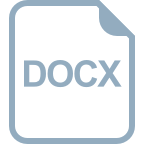
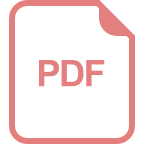
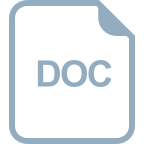
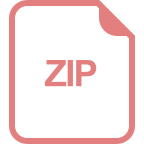
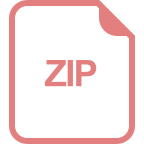
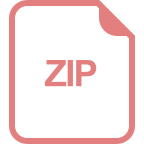
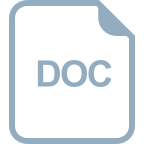
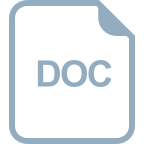
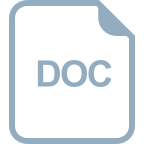
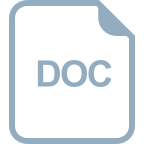
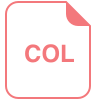
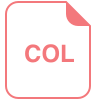