python绘制双柱状图
时间: 2023-07-08 12:53:49 浏览: 107
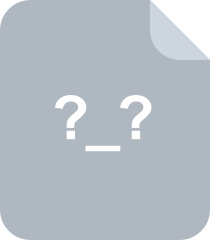
基于python的柱状图绘制与实现
好的,可以使用Python中的Matplotlib库来绘制双柱状图。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 数据
labels = ['A', 'B', 'C', 'D', 'E']
men_means = [20, 35, 30, 35, 27]
women_means = [25, 32, 34, 20, 25]
x = np.arange(len(labels))
width = 0.35
# 绘图
fig, ax = plt.subplots()
rects1 = ax.bar(x - width/2, men_means, width, label='Men')
rects2 = ax.bar(x + width/2, women_means, width, label='Women')
# 添加轴标签、标题和图例
ax.set_ylabel('Scores')
ax.set_xlabel('Groups')
ax.set_title('Scores by group and gender')
ax.set_xticks(x)
ax.set_xticklabels(labels)
ax.legend()
# 添加数据标签
def autolabel(rects):
for rect in rects:
height = rect.get_height()
ax.annotate('{}'.format(height),
xy=(rect.get_x() + rect.get_width() / 2, height),
xytext=(0, 3), # 3 points vertical offset
textcoords="offset points",
ha='center', va='bottom')
autolabel(rects1)
autolabel(rects2)
plt.show()
```
解释一下这段代码:
1. 导入必要的库:Matplotlib和NumPy。
2. 准备数据:这里我们有两组数据,每组数据都有5个元素,分别代表5个组的男女得分。
3. 创建x轴坐标和柱状图的宽度。
4. 使用Matplotlib的subplots()函数创建一个图形对象和一个轴对象。
5. 使用Matplotlib的bar()函数在轴对象上创建柱状图。
6. 添加轴标签、标题和图例。
7. 添加数据标签:这里使用Matplotlib的annotate()函数添加每个柱状图的数值标签。
8. 显示图形。
运行这段代码,你会看到一个双柱状图,其中每个组都有男女两种得分。
阅读全文
相关推荐
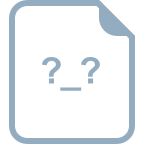














