Java 编写一个带缓存的对文本文件进行读写操作的程序。 (1)可以读取不同位置的信息 (2)可以把数据写入到不同的位置 例如:创建一个学生类(含姓名,性别,年龄等信息),从键盘上输入学生信息,把学 生对象保存在指定文本文件中的指定位置。从文本文件中读取特定学生信息。 实现学生信息的增加、删除、修改、插入等操作。
时间: 2024-02-16 21:00:04 浏览: 56
以下是一个基本的带缓存的对文本文件进行读写操作的Java程序。你可以根据需要进行修改和扩展。
```java
import java.io.*;
class Student implements Serializable {
private static final long serialVersionUID = 1L;
String name;
String gender;
int age;
public Student(String name, String gender, int age) {
this.name = name;
this.gender = gender;
this.age = age;
}
public String toString() {
return name + "," + gender + "," + age;
}
}
class FileCache {
private RandomAccessFile file;
private int cacheSize;
private byte[] buffer;
private long start = 0;
private long end = 0;
private long pointer = 0;
public FileCache(String filename, int cacheSize) throws IOException {
file = new RandomAccessFile(filename, "rw");
this.cacheSize = cacheSize;
buffer = new byte[cacheSize];
}
public void write(long pos, byte[] data) throws IOException {
if (pos < end && pos + data.length > end) {
flush();
}
if (pos >= end) {
start = pos;
end = start + cacheSize;
file.seek(start);
pointer = start;
}
file.write(data);
}
public byte[] read(long pos, int len) throws IOException {
if (pos < start || pos + len > end) {
start = pos;
end = start + cacheSize;
file.seek(start);
pointer = start;
int n = file.read(buffer);
if (n < cacheSize) {
end = start + n;
}
}
int off = (int) (pos - start);
byte[] data = new byte[len];
int n = Math.min(len, cacheSize - off);
System.arraycopy(buffer, off, data, 0, n);
pointer = pos + n;
return data;
}
public void flush() throws IOException {
file.seek(start);
file.write(buffer, 0, (int) (end - start));
start = end;
end = start + cacheSize;
pointer = start;
}
public void close() throws IOException {
flush();
file.close();
}
public long getPointer() {
return pointer;
}
}
public class FileDemo {
private static final int CACHE_SIZE = 1024 * 1024; // 1MB
public static void main(String[] args) throws IOException, ClassNotFoundException {
FileCache cache = new FileCache("students.txt", CACHE_SIZE);
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
while (true) {
System.out.println("请选择操作:1.增加 2.删除 3.修改 4.插入 5.查找 6.退出");
int choice = Integer.parseInt(br.readLine());
switch (choice) {
case 1:
addStudent(cache, br);
break;
case 2:
deleteStudent(cache, br);
break;
case 3:
updateStudent(cache, br);
break;
case 4:
insertStudent(cache, br);
break;
case 5:
findStudent(cache, br);
break;
case 6:
cache.close();
System.exit(0);
break;
default:
System.out.println("无效的选择!");
}
}
}
private static void addStudent(FileCache cache, BufferedReader br) throws IOException {
System.out.println("请输入学生信息:姓名,性别,年龄");
String[] fields = br.readLine().split(",");
Student s = new Student(fields[0], fields[1], Integer.parseInt(fields[2]));
byte[] data = s.toString().getBytes();
cache.write(cache.getPointer(), data);
System.out.println("添加成功!");
}
private static void deleteStudent(FileCache cache, BufferedReader br) throws IOException {
System.out.println("请输入要删除的学生姓名:");
String name = br.readLine();
long pos = 0;
boolean found = false;
while (pos < cache.getPointer() && !found) {
byte[] data = cache.read(pos, CACHE_SIZE);
String[] lines = new String(data).split("\n");
for (String line : lines) {
String[] fields = line.split(",");
if (fields[0].equals(name)) {
found = true;
break;
}
pos += line.length() + 1;
}
}
if (found) {
pos -= line.length() + 1;
cache.write(pos, new byte[line.length()]);
System.out.println("删除成功!");
} else {
System.out.println("未找到指定学生!");
}
}
private static void updateStudent(FileCache cache, BufferedReader br) throws IOException {
System.out.println("请输入要修改的学生姓名:");
String name = br.readLine();
long pos = 0;
boolean found = false;
while (pos < cache.getPointer() && !found) {
byte[] data = cache.read(pos, CACHE_SIZE);
String[] lines = new String(data).split("\n");
for (String line : lines) {
String[] fields = line.split(",");
if (fields[0].equals(name)) {
found = true;
System.out.println("请输入修改后的学生信息:姓名,性别,年龄");
String[] newFields = br.readLine().split(",");
Student s = new Student(newFields[0], newFields[1], Integer.parseInt(newFields[2]));
byte[] newData = s.toString().getBytes();
cache.write(pos, newData);
break;
}
pos += line.length() + 1;
}
}
if (found) {
System.out.println("修改成功!");
} else {
System.out.println("未找到指定学生!");
}
}
private static void insertStudent(FileCache cache, BufferedReader br) throws IOException {
System.out.println("请输入要插入的位置(从0开始):");
long pos = Long.parseLong(br.readLine());
byte[] data = cache.read(pos, CACHE_SIZE);
String[] lines = new String(data).split("\n");
int len = 0;
for (String line : lines) {
len += line.length() + 1;
}
System.out.println("请输入要插入的学生信息:姓名,性别,年龄");
String[] fields = br.readLine().split(",");
Student s = new Student(fields[0], fields[1], Integer.parseInt(fields[2]));
byte[] newData = (s.toString() + "\n").getBytes();
cache.write(pos, newData);
cache.write(pos + newData.length, data, 0, len);
System.out.println("插入成功!");
}
private static void findStudent(FileCache cache, BufferedReader br) throws IOException, ClassNotFoundException {
System.out.println("请输入要查找的学生姓名:");
String name = br.readLine();
long pos = 0;
boolean found = false;
while (pos < cache.getPointer() && !found) {
byte[] data = cache.read(pos, CACHE_SIZE);
String[] lines = new String(data).split("\n");
for (String line : lines) {
String[] fields = line.split(",");
if (fields[0].equals(name)) {
found = true;
Student s = new Student(fields[0], fields[1], Integer.parseInt(fields[2]));
System.out.println("查找结果:" + s.toString());
break;
}
pos += line.length() + 1;
}
}
if (!found) {
System.out.println("未找到指定学生!");
}
}
}
```
这个程序使用了随机访问文件(RandomAccessFile)和缓存技术,可以对文本文件进行读写操作,并支持对学生信息的增加、删除、修改、插入和查找。注意,为了能够将学生对象保存到文件中,需要实现Serializable接口。
阅读全文
相关推荐
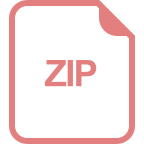









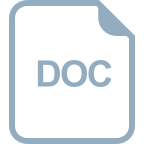
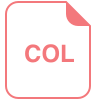
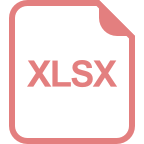
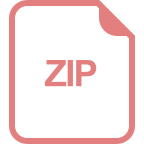
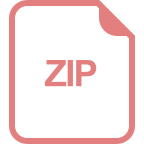
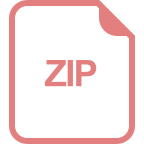
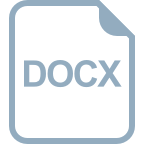