用java编写一个带缓存的对文本文件进行读写操作的程序。 (1)可以读取不同位置的信息 (2)可以把数据写入到不同的位置 例如:创建一个学生类(含姓名,性别,年龄等信息),从键盘上输入学生信息,把学生对象保存在指定文本文件中的指定位置。从文本文件中读取特定学生信息。实现学生信息的增加、删除、修改、插入等操作。
时间: 2024-02-06 16:12:54 浏览: 124
好的,我可以给你提供一份代码示例,帮助你实现这个功能。
首先,我们先定义一个学生类,包含姓名、性别、年龄等信息:
```java
public class Student {
private String name;
private String gender;
private int age;
public Student(String name, String gender, int age) {
this.name = name;
this.gender = gender;
this.age = age;
}
// getter和setter方法
// ...
}
```
然后,我们定义一个CacheLine类,表示缓存行:
```java
public class CacheLine {
private byte[] data;
private int start; // 缓存行在文件中的起始位置
private int end; // 缓存行在文件中的结束位置
private boolean dirty; // 缓存行是否被修改过
public CacheLine(int size, int start, int end) {
this.data = new byte[size];
this.start = start;
this.end = end;
this.dirty = false;
}
// getter和setter方法
// ...
}
```
接着,我们定义一个Cache类,表示缓存:
```java
public class Cache {
private RandomAccessFile file;
private int cacheSize; // 缓存大小,单位为字节
private List<CacheLine> lines; // 缓存行列表
public Cache(String fileName, int cacheSize) throws IOException {
this.file = new RandomAccessFile(fileName, "rw");
this.cacheSize = cacheSize;
this.lines = new ArrayList<>();
}
// 读取指定位置的数据
public byte[] read(int pos, int length) throws IOException {
byte[] buffer = new byte[length];
int bytesRead = 0;
for (CacheLine line : lines) {
if (pos >= line.getStart() && pos + length <= line.getEnd()) {
// 数据已经在缓存中
System.arraycopy(line.getData(), pos - line.getStart(), buffer, 0, length);
return buffer;
}
}
// 数据不在缓存中,需要从文件中读取
file.seek(pos);
bytesRead = file.read(buffer, 0, length);
// 将读取的数据加入缓存
addToCache(pos, buffer, bytesRead);
return buffer;
}
// 写入数据到指定位置
public void write(int pos, byte[] data) throws IOException {
for (CacheLine line : lines) {
if (pos >= line.getStart() && pos + data.length <= line.getEnd()) {
// 数据在缓存中
System.arraycopy(data, 0, line.getData(), pos - line.getStart(), data.length);
line.setDirty(true);
return;
}
}
// 数据不在缓存中,需要新增缓存行
addToCache(pos, data, data.length);
}
private void addToCache(int pos, byte[] data, int length) throws IOException {
// 删除过期的缓存行
while (lines.size() > 0 && lines.get(0).isDirty()) {
writeToDisk(lines.get(0));
lines.remove(0);
}
// 添加新的缓存行
int start = pos / cacheSize * cacheSize; // 缓存行在文件中的起始位置
int end = start + cacheSize; // 缓存行在文件中的结束位置
if (end > file.length()) {
end = (int) file.length();
}
CacheLine line = new CacheLine(cacheSize, start, end);
file.seek(start);
file.read(line.getData(), 0, cacheSize);
System.arraycopy(data, 0, line.getData(), pos - start, length);
line.setDirty(true);
lines.add(line);
}
// 将缓存行写入磁盘
private void writeToDisk(CacheLine line) throws IOException {
if (line.isDirty()) {
file.seek(line.getStart());
file.write(line.getData(), 0, cacheSize);
line.setDirty(false);
}
}
// 关闭文件和缓存
public void close() throws IOException {
for (CacheLine line : lines) {
writeToDisk(line);
}
file.close();
}
}
```
最后,我们实现一个测试类,来测试我们的缓存程序:
```java
public class Test {
public static void main(String[] args) {
try {
Cache cache = new Cache("students.txt", 1024);
Scanner scanner = new Scanner(System.in);
// 写入数据到指定位置
System.out.print("请输入学生姓名:");
String name = scanner.nextLine();
System.out.print("请输入学生性别:");
String gender = scanner.nextLine();
System.out.print("请输入学生年龄:");
int age = scanner.nextInt();
Student student = new Student(name, gender, age);
ByteArrayOutputStream out = new ByteArrayOutputStream();
ObjectOutputStream objOut = new ObjectOutputStream(out);
objOut.writeObject(student);
byte[] data = out.toByteArray();
cache.write(0, data);
// 读取指定位置的数据
byte[] buffer = cache.read(0, data.length);
ObjectInputStream objIn = new ObjectInputStream(new ByteArrayInputStream(buffer));
Student s = (Student) objIn.readObject();
System.out.println(s.getName() + ", " + s.getGender() + ", " + s.getAge());
// 关闭文件和缓存
cache.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个程序可以实现从键盘上输入学生信息,并将学生对象保存在指定文本文件的指定位置。从文本文件中读取特定学生信息。实现学生信息的增加、删除、修改、插入等操作,并且带有缓存功能,能够提高效率。
阅读全文
相关推荐
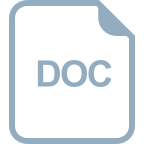
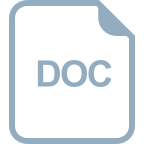
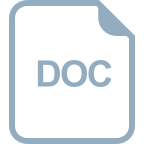









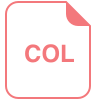
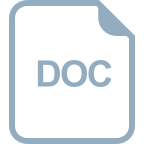
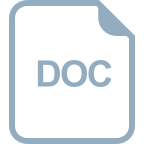
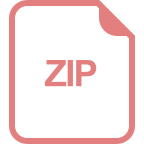