编写一个带缓存的对文本文件进行读写操作的java程序。 (1)可以读取不同位置的信息 (2)可以把数据写入到不同的位置
时间: 2024-02-06 22:11:24 浏览: 68
好的,我可以为您提供一个简单的带缓存的对文本文件进行读写操作的Java程序。具体实现如下:
```java
import java.io.*;
public class FileReadWrite {
private static final int BUFFER_SIZE = 1024; // 缓存大小为1024字节
private byte[] buffer = new byte[BUFFER_SIZE];
private File file;
private RandomAccessFile raf;
public FileReadWrite(String filePath) throws IOException {
file = new File(filePath);
if (!file.exists()) {
file.createNewFile();
}
raf = new RandomAccessFile(file, "rw");
}
// 读取指定位置的信息
public String read(int position, int length) throws IOException {
raf.seek(position); // 定位到指定位置
int readLength = 0;
StringBuilder sb = new StringBuilder();
while (readLength < length && raf.read(buffer) != -1) {
int len = Math.min(length - readLength, BUFFER_SIZE); // 读取数据的长度
sb.append(new String(buffer, 0, len)); // 将读取的数据追加到StringBuilder中
readLength += len;
}
return sb.toString();
}
// 写入指定位置的信息
public void write(int position, String content) throws IOException {
raf.seek(position); // 定位到指定位置
byte[] bytes = content.getBytes();
int writeLength = 0;
while (writeLength < bytes.length) {
int len = Math.min(bytes.length - writeLength, BUFFER_SIZE); // 写入数据的长度
raf.write(bytes, writeLength, len); // 写入数据
writeLength += len;
}
}
// 关闭文件流
public void close() throws IOException {
raf.close();
}
public static void main(String[] args) throws IOException {
FileReadWrite fileRW = new FileReadWrite("test.txt");
fileRW.write(0, "Hello, world!"); // 写入数据到文件的开头
System.out.println(fileRW.read(0, 5)); // 读取文件开头的5个字符
fileRW.write(7, "Java"); // 写入数据到文件的第8个位置
System.out.println(fileRW.read(0, 12)); // 读取文件前12个字符
fileRW.close(); // 关闭文件流
}
}
```
这个程序实现了带缓存的对文本文件进行读写操作,可以读取不同位置的信息,也可以把数据写入到不同的位置。程序中的read方法和write方法分别实现了读取指定位置的信息和写入指定位置的信息的功能。在main方法中,我们测试了程序的读写功能,可以看到程序可以正确地读取和写入文件的数据。
阅读全文
相关推荐
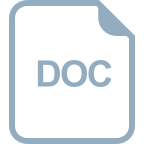
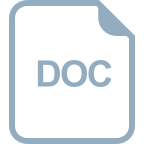
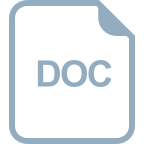









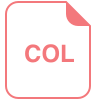
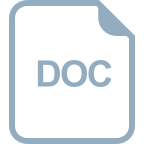
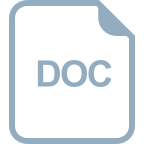
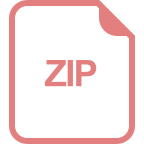
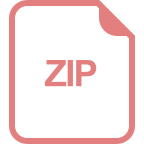
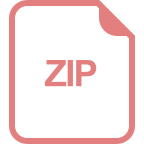
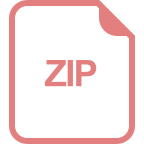