TypeError: Value after * must be an iterable, not float
时间: 2024-05-03 13:19:22 浏览: 428
这个错误通常发生在使用 * 运算符时,后面的值不是可迭代的对象而是一个浮点数。以下是一个示例:
```
numbers = [1, 2, 3]
result = [*numbers, 4.5]
print(result)
```
这段代码中,我们试图将一个浮点数添加到列表 `numbers` 中,并使用 * 运算符将整个列表展开为一个新的列表。但是,由于浮点数不是可迭代的对象,所以会抛出 TypeError 错误。
要解决这个问题,我们需要确保 * 运算符后面的值是一个可迭代的对象。可以将浮点数放入一个列表中,然后将其与其他可迭代的对象一起展开:
```
numbers = [1, 2, 3]
result = [*numbers, *[4.5]]
print(result)
```
这段代码中,我们将浮点数 4.5 放入一个列表中,然后使用 * 运算符展开整个列表。这样就能够避免 TypeError 错误。
相关问题
torchsummary TypeError: Value after * must be an iterable, not int
这个错误通常发生在使用`torchsummary`库时,当尝试将一个整数作为参数传递给`torchsummary.summary`函数时会出现这个错误。`torchsummary.summary`函数期望接收一个模型实例和输入张量的大小作为参数。
请确保你正确地传递了模型实例和输入张量的大小。例如,你可以这样使用`torchsummary.summary`函数:
```python
import torch
from torchsummary import summary
model = YourModel()
input_size = (3, 224, 224) # 输入张量的大小
summary(model, input_size)
```
请将`YourModel`替换为你的实际模型类,并根据你的输入张量大小进行相应的更改。如果问题仍然存在,请提供更多的代码和错误堆栈跟踪,以便我可以更好地帮助你解决问题。
TypeError: Value after * must be an iterable, not numpy.uint8
This error occurs when you try to multiply a single value (which is not iterable) with a numpy array. To fix this error, you need to ensure that both operands of the multiplication operation are iterable.
For example, if you have a numpy array `a` and a scalar value `b`, you can multiply them using the following code:
```
import numpy as np
a = np.array([1, 2, 3])
b = 2
result = a * b
```
In this example, `b` is a scalar value, but it is automatically broadcasted to match the shape of `a` (which is `[1, 2, 3]`). This means that `b` is effectively converted to the array `[2, 2, 2]`, and then multiplied element-wise with `a` to produce the result `[2, 4, 6]`.
If you still get the same error, make sure that the data type of your numpy array is compatible with the scalar value you want to use for multiplication. For example, if your numpy array has a data type of `uint8`, you should use a scalar value that can be converted to `uint8`, such as an integer between 0 and 255.
阅读全文
相关推荐
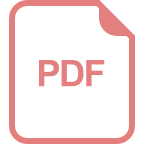
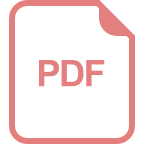
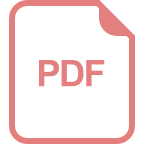













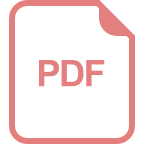
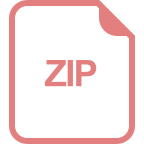