用选择法排序法对 10 个整数排序。10 个整数用 scan 函数输入
时间: 2023-06-25 15:07:09 浏览: 52
下面是使用选择排序法对10个整数进行排序的示例代码:
```c
#include <stdio.h>
void selectionSort(int arr[], int n);
int main() {
int arr[10];
int i;
printf("请输入10个整数:\n");
for (i = 0; i < 10; i++) {
scanf("%d", &arr[i]);
}
selectionSort(arr, 10);
printf("排序后的结果为:\n");
for (i = 0; i < 10; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
void selectionSort(int arr[], int n) {
int i, j, minIndex, temp;
for (i = 0; i < n - 1; i++) {
minIndex = i;
for (j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
if (minIndex != i) {
temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
}
```
这段代码首先通过 `scanf` 函数从用户输入中读取10个整数,然后调用 `selectionSort` 函数进行排序,最后输出排序后的结果。 `selectionSort` 函数实现了选择排序算法,每次找到未排序部分中最小的元素,并将其交换到已排序部分的最后。
注意,选择排序算法的时间复杂度为 $O(n^2)$,对于大规模数据的排序可能会比较慢。如果需要排序大量数据,可以使用更高效的排序算法,比如快速排序或归并排序。
相关推荐
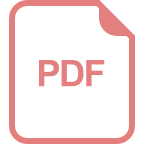














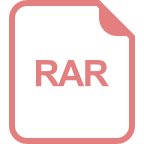