map 的key值模糊查询
时间: 2024-01-25 20:04:38 浏览: 25
在 map 中进行 key 值的模糊查询,可以使用 C++ STL 中的 lower_bound 和 upper_bound 函数结合字符串比较函数来实现。具体步骤如下:
1. 定义一个字符串比较函数,用于判断两个字符串的大小关系,可以使用 C++ STL 中的 string 类的 compare 函数。
2. 使用 lower_bound 函数查找第一个大于等于目标字符串的位置。
3. 使用 upper_bound 函数查找第一个大于目标字符串的位置。
4. 遍历 [lower_bound, upper_bound) 区间内的所有键值对,并判断键是否符合模糊查询的条件。
下面是一个示例代码,使用前需要自定义字符串比较函数 cmp:
```c++
#include <iostream>
#include <map>
#include <string>
using namespace std;
int cmp(const string& a, const string& b) {
// 自定义字符串比较函数,返回值表示 a 是否小于 b
// 返回值 < 0,表示 a < b
// 返回值 = 0,表示 a == b
// 返回值 > 0,表示 a > b
return a.compare(0, b.length(), b);
}
int main() {
map<string, int> mp;
mp["apple"] = 1;
mp["banana"] = 2;
mp["cherry"] = 3;
mp["orange"] = 4;
mp["peach"] = 5;
mp["pear"] = 6;
string target = "p"; // 模糊查询的目标字符串
auto iter_lb = lower_bound(mp.begin(), mp.end(), target, cmp); // 查找第一个大于等于目标字符串的位置
auto iter_ub = upper_bound(mp.begin(), mp.end(), target, cmp); // 查找第一个大于目标字符串的位置
for (auto iter = iter_lb; iter != iter_ub; iter++) {
if (iter->first.find(target) != string::npos) { // 判断键是否符合模糊查询的条件
cout << iter->first << " " << iter->second << endl;
}
}
return 0;
}
```
输出结果为:
```
peach 5
pear 6
```
相关推荐
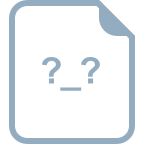














