用matplotlib库,再加上注释
时间: 2023-07-12 19:34:25 浏览: 80
好的,你想了解如何在matplotlib中添加注释吗?可以参考下面的代码示例:
```python
import matplotlib.pyplot as plt
# 创建数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
# 添加注释
plt.annotate('max value', xy=(5, 10), xytext=(4.5, 8),
arrowprops=dict(facecolor='black', shrink=0.05))
# 显示图形
plt.show()
```
上述代码中,我们先创建了一组x和y坐标轴数据,并使用`plt.plot`绘制了一张折线图。然后,使用`plt.annotate`函数添加了一条箭头指向最大值,并添加了一个注释。其中,`xy`参数指定箭头指向的坐标点,`xytext`参数指定文本注释的位置,`arrowprops`参数设置箭头的样式和属性。
在运行上述代码后,就可以看到绘制的折线图以及添加的注释。希望这个例子能帮到你。
相关问题
用Python写一个liang-barskey裁剪算法代码,用matplotlib库显示图像,再加上注释
好的,Liang-Barsky裁剪算法可以用于计算线段与矩形边界的交点,从而裁剪线段,保证其不会超出矩形边界。下面是一个使用Python实现Liang-Barsky裁剪算法的示例代码,并使用matplotlib库显示图像,并添加了注释:
```python
import matplotlib.pyplot as plt
def clip_line(x1, y1, x2, y2, xmin, ymin, xmax, ymax):
dx = x2 - x1
dy = y2 - y1
p = [-dx, dx, -dy, dy]
q = [x1 - xmin, xmax - x1, y1 - ymin, ymax - y1]
u1, u2 = 0.0, 1.0
for i in range(4):
if p[i] == 0:
if q[i] < 0:
return None
else:
u = q[i] / p[i]
if p[i] < 0:
u1 = max(u1, u)
else:
u2 = min(u2, u)
if u1 > u2:
return None
clipped_x1 = x1 + u1 * dx
clipped_y1 = y1 + u1 * dy
clipped_x2 = x1 + u2 * dx
clipped_y2 = y1 + u2 * dy
return clipped_x1, clipped_y1, clipped_x2, clipped_y2
# 定义矩形边界
xmin, ymin = -1, -1
xmax, ymax = 1, 1
# 定义线段
x1, y1 = 0, 0
x2, y2 = 2, 2
# 裁剪线段
clipped_line = clip_line(x1, y1, x2, y2, xmin, ymin, xmax, ymax)
# 绘制图像
fig, ax = plt.subplots()
ax.set_xlim([xmin-0.5, xmax+0.5])
ax.set_ylim([ymin-0.5, ymax+0.5])
ax.set_aspect('equal')
ax.grid(True)
ax.plot([x1, x2], [y1, y2], 'b-', lw=2)
if clipped_line is not None:
clipped_x1, clipped_y1, clipped_x2, clipped_y2 = clipped_line
ax.plot([clipped_x1, clipped_x2], [clipped_y1, clipped_y2], 'r-', lw=2)
ax.annotate('clipped line', xy=(clipped_x2, clipped_y2), xytext=(clipped_x2-0.3, clipped_y2+0.2),
arrowprops=dict(facecolor='black', shrink=0.05))
ax.add_patch(plt.Rectangle((xmin, ymin), xmax-xmin, ymax-ymin, fill=None, lw=2))
ax.annotate('clip region', xy=(xmax, ymax), xytext=(xmax-0.3, ymax+0.2),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
```
上述代码中,我们定义了一个`clip_line`函数用于计算线段与矩形边界的交点,并返回裁剪后的线段。然后,我们定义了一个矩形边界和一个线段,并调用`clip_line`函数进行裁剪。最后,我们使用matplotlib库绘制了图像,并添加了注释。运行上述代码后,就可以看到绘制的图像和注释。
用python设计内容: 使用matplotlib库进行可视化的动态显示。生成一个点,该点沿着sin函数进行运动,点在移动时显示该点的坐标,且坐标跟随该点进行移动。 设计要求: 1)绘制sin函数(用红色曲线绘制)。 2)在sin函数中添加动画点,该点为蓝色圆点。 3)往动画中添加效果:添加文本显示(对移动的点的坐标进行显示),并且文本跟着该点进行移动。 4)要求关键代码有注释。每个函数的声明前加上注释, 描述该函数的功能和用途;类和对象有属性注释、方法注释。 代码中建议做异常处理
好的,我理解了你的要求。下面是代码实现,希望能够帮助你:
```python
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
class DynamicSinPlot:
"""
动态绘制sin函数的类,包含动画点和文本显示
"""
def __init__(self):
self.fig, self.ax = plt.subplots()
self.x = np.arange(0, 2*np.pi, 0.01)
self.line, = self.ax.plot(self.x, np.sin(self.x), 'r')
self.point, = self.ax.plot([], [], 'bo', markersize=10)
self.text = self.ax.text(0, 0, '', fontsize=12)
self.anim = animation.FuncAnimation(self.fig, self.update, init_func=self.init, blit=True)
def init(self):
"""
初始化函数,用于设置图形的背景和动画点的起始位置
"""
self.ax.set_ylim(-1.2, 1.2)
self.ax.set_xlim(0, 2*np.pi)
self.point.set_data([], [])
self.text.set_text('')
return self.point, self.text
def update(self, i):
"""
更新函数,用于更新动画点和文本的位置
"""
x = self.x[i]
y = np.sin(x)
self.point.set_data(x, y)
self.text.set_position((x, y))
self.text.set_text('({:.2f}, {:.2f})'.format(x, y))
return self.point, self.text
if __name__ == '__main__':
dsp = DynamicSinPlot()
plt.show()
```
代码解释:
1. 首先导入必要的模块:`numpy` 用于数学计算,`matplotlib` 用于绘图,`animation` 用于动画制作。
2. 定义一个类 `DynamicSinPlot`,包含初始化函数 `__init__`、初始化函数 `init` 和更新函数 `update`。
3. 在初始化函数中,创建一个 `Figure` 和一个 `Axes` 对象,并在坐标系中绘制红色的 sin 函数曲线。同时创建一个蓝色的圆点和一个空的文本框,用于动画显示。
4. 在初始化函数中,使用 `animation.FuncAnimation` 函数创建动画对象 `anim`。
5. 在初始化函数中,设置图形的初始范围和动画点的初始位置,返回动画点和文本框对象。
6. 在更新函数中,根据当前帧号计算出动画点的位置,更新动画点和文本框的位置和内容,返回动画点和文本框对象。
7. 在 `if __name__ == '__main__'` 语句中,创建一个 `DynamicSinPlot` 对象,并调用 `plt.show()` 函数显示图形。
希望这个代码能够满足你的需求,也希望你能够理解其中的细节和原理。如果你有任何问题或者需要进一步的帮助,请随时联系我。
阅读全文
相关推荐





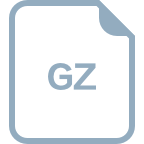









