List<Long> documentIdList = documentList.stream().map(Document::getCateId).collect(Collectors.toList());
时间: 2024-04-11 09:32:36 浏览: 83
这段代码是用来将一个 `documentList` 中的所有 `Document` 对象的 `cateId` 属性提取出来,并存储在一个 `List<Long>` 类型的变量 `documentIdList` 中。这里使用了 Java 8 的 Stream API 中的 `map()` 方法和 `collect()` 方法来实现。
具体来说,`stream()` 方法将 `documentList` 转换为一个流,然后调用 `map()` 方法将每个 `Document` 对象映射为其 `cateId` 属性值。最后,`collect()` 方法将映射结果收集到一个新的列表中。
请注意,这里假设 `Document` 类中有一个名为 `getCateId()` 的方法,用于获取该对象的 `cateId` 属性值。
相关问题
java中,我有一个list,里面都是Document,我想对Document中的一个字段进行排序
可以使用Java 8的Stream API配合Comparator来对List中的Document对象的某个字段进行排序。假设你的List中存储的是Document对象,你想要按照Document对象中的某个字段fieldName进行排序,可以按以下步骤操作:
1. 使用stream方法获取一个Stream对象。
```
Stream<Document> documentStream = documentList.stream();
```
2. 使用sorted方法对Stream中的元素进行排序,传入Comparator对象作为参数。
```
Comparator<Document> comparator = (d1, d2) -> d1.getString(fieldName).compareTo(d2.getString(fieldName));
Stream<Document> sortedDocumentStream = documentStream.sorted(comparator);
```
上面的代码中,我们通过Comparator对象传入了一个比较器,这个比较器比较的是Document对象中的fieldName字段的值。
3. 使用collect方法将排序后的Stream对象转换为List对象。
```
List<Document> sortedDocumentList = sortedDocumentStream.collect(Collectors.toList());
```
这样,你就可以按照Document对象中的某个字段进行排序,并将排序后的结果存储在List对象中了。如果你想要按照其他字段进行排序,只需要修改Comparator对象的比较逻辑即可。
foreach not applicable to type 'org.jsoup.select.Elements'
如果你在使用 `for-each` 循环来遍历 `Elements` 对象时遇到了 `foreach not applicable to type 'org.jsoup.select.Elements'` 错误,那么可能是因为 `Elements` 对象不支持 `for-each` 循环。解决方法是将 `Elements` 对象转换为 `List<Element>` 类型,然后再使用 `for-each` 循环遍历。以下是一个示例代码:
```java
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
public class HtmlParser {
public static void main(String[] args) {
String html = "<html><body><p>This is a paragraph</p><table><tr><td>Table cell</td></tr></table></body></html>";
Document doc = Jsoup.parse(html);
Element body = doc.body();
// 获取所有文本节点
Elements textNodes = body.select(":not(table) > *").not("table *").not("table");
// 将Elements对象转换为List类型
List<Element> textNodeList = textNodes.stream().collect(Collectors.toList());
// 遍历List
for (Element textNode : textNodeList) {
System.out.println(textNode.text());
}
}
}
```
在上述代码中,我们将 `Elements` 对象通过 `stream()` 方法转换为 `Stream<Element>` 对象,然后使用 `collect(Collectors.toList())` 方法将 `Stream<Element>` 对象转换为 `List<Element>` 对象。最后,我们使用 `for-each` 循环遍历 `List<Element>` 对象。
阅读全文
相关推荐
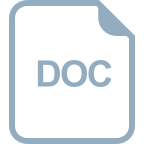
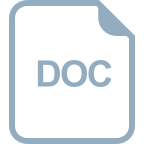
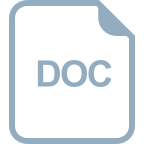
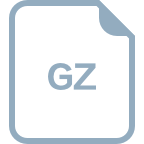
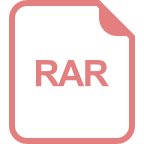
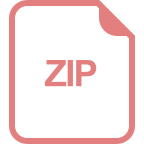
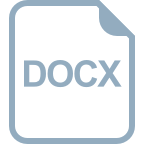
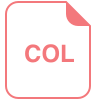
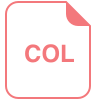
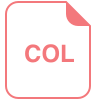
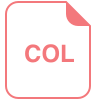
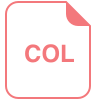
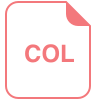
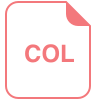


