qt使用tcp实现客户端与服务器交互数据具体案例
时间: 2024-10-21 22:06:34 浏览: 28
在Qt中,使用TCP套接字(QTcpSocket)可以创建客户端和服务器,用于实现实时的数据交换。下面是一个简单的例子,展示如何在Qt中建立一个TCP客户端和服务器:
**服务器端示例(server.cpp)**:
```cpp
#include <QTcpServer>
#include <QDebug>
class Server : public QTcpServer
{
public:
voidincomingConnection(qintptr socketDescriptor)
{
QByteArray msg = "Welcome to the server!";
QDataStream out(&socketDescriptor, QIODevice::WriteOnly);
out << msg;
qDebug() << "Connected client with descriptor:" << socketDescriptor;
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Server server;
if (server.listen(QHostAddress::Any, 8000)) // Listen on any IP and port 8000
{
qDebug() << "Server is listening.";
while (!server.isListening()) {} // Wait for a connection
return app.exec();
}
else
{
qWarning() << "Failed to start server.";
return -1;
}
}
```
在这个例子中,服务器监听8000端口,并在连接请求时向新连接的客户端发送欢迎消息。
**客户端示例(client.cpp)**:
```cpp
#include <QTcpSocket>
#include <QApplication>
#include <QMessageBox>
class Client
{
public:
voidconnectToServer()
{
QTcpSocket* client = new QTcpSocket(this);
client->connectToHost("localhost", 8000); // Replace with your server's address
connect(client, &QTcpSocket::connected, this, [client] { client->send("Hello from client!"); });
connect(client, &QTcpSocket::readyRead, this, [client] {
QByteArray data;
client->readAll(data);
QMessageBox::information(this, "Message", QString::fromStdString(data.toStdString()));
});
connect(client, &QTcpSocket::disconnected, this, &Client::onDisconnected);
}
private:
void onDisconnected()
{
QMessageBox::information(this, "Connection Lost", "The connection was closed.");
}
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Client client;
client.connectToServer();
return app.exec();
}
```
客户端尝试连接到服务器,一旦连接成功,它会发送一条消息并接收来自服务器的响应。如果连接关闭,会显示相应提示。
阅读全文
相关推荐
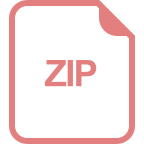
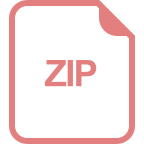
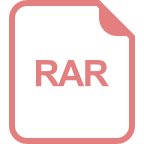
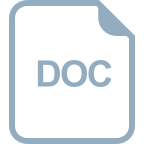
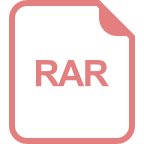
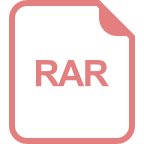
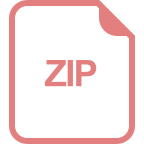
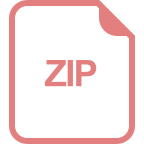
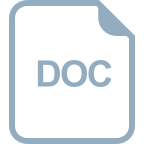
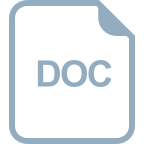
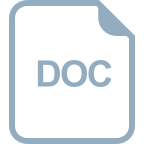
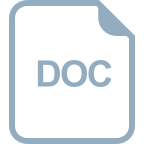
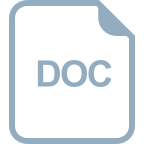
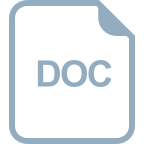
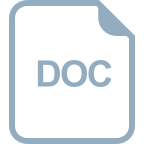
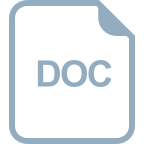
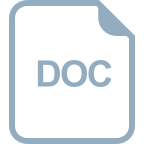
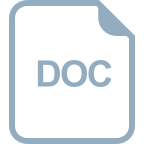
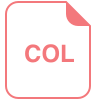