用c语言输入多个字符串,输出最大字符串和最长字符串
时间: 2024-12-10 18:07:34 浏览: 26
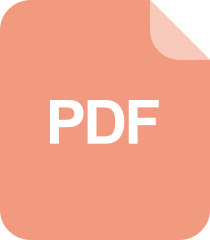
C语言求两个字符串的最长公共子串

在C语言中,可以使用循环结构(如for或while)以及动态内存分配函数`malloc()`来输入多个字符串并找出其中的最大字符串和最长字符串。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STR_LENGTH 100 // 最大字符串长度假设为100
void inputAndFindMaxAndLongest(char** strArray, int count) {
char* maxStr = NULL;
size_t maxLength = 0;
for (int i = 0; i < count; ++i) {
char* tempStr = malloc(MAX_STR_LENGTH * sizeof(char)); // 动态分配内存
if (!tempStr) {
perror("Memory allocation failed");
return;
}
printf("Enter string %d: ", i + 1);
fgets(tempStr, MAX_STR_LENGTH, stdin); // 输入字符串,包括换行符
// 移除fgets结尾的换行符
tempStr[strlen(tempStr) - 1] = '\0';
if (strlen(tempStr) > maxLength) {
maxLength = strlen(tempStr);
maxStr = tempStr;
}
free(tempStr); // 释放内存
}
if (maxStr) {
printf("The longest string is '%s', length: %zu\n", maxStr, maxLength);
free(maxStr); // 再次释放最大字符串的内存
} else {
printf("No strings were entered.\n");
}
}
int main() {
int nStrings;
printf("How many strings do you want to enter? ");
scanf("%d", &nStrings);
char** strArray = (char**)malloc(nStrings * sizeof(char*));
if (!strArray) {
perror("Memory allocation failed");
return 1;
}
inputAndFindMaxAndLongest(strArray, nStrings);
for (int i = 0; i < nStrings; ++i) {
free(strArray[i]); // 可选:释放每个单独的字符串内存,这里为了简洁略去
}
free(strArray); // 释放数组的内存
return 0;
}
```
在这个程序中,用户会被询问想要输入多少个字符串,然后依次输入。程序会记录下最长的那个字符串及其长度,并在结束后将其打印出来。
阅读全文
相关推荐
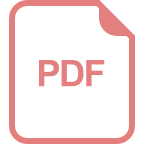
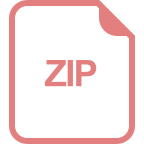
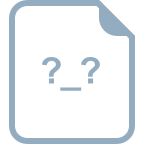
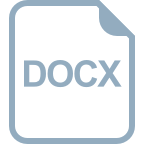
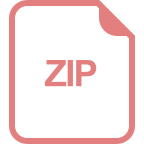
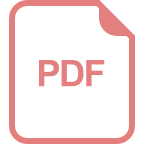
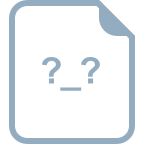
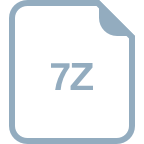
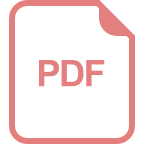
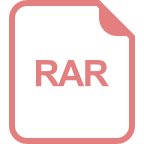
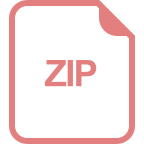
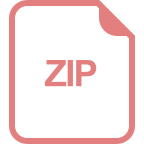
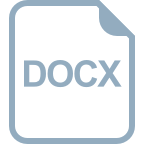
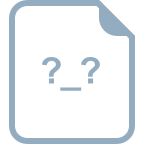
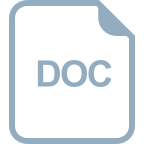

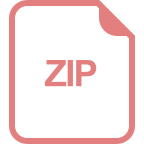
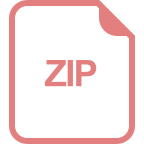