用vhdl设计一个带有清零、使能的分频器,输出以下频率:0.5Hz,1hz,2hz,并用led灯显示出来。
时间: 2024-12-16 15:18:55 浏览: 26
在 VHDL 设计中,创建一个带清零、使能功能的分频器通常涉及以下几个步骤:
1. **模块定义**:
首先,你需要定义一个模块(entity),如 "Counter_FrequencyDivider",其中包含输入信号如 `Enable`, `Reset` 和定时输入 `InputClock`。
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity Counter_FrequencyDivider is
Port (
Enable : in std_logic; -- 使能信号
Reset : in std_logic; -- 清零信号
InputClock : in std_logic_vector(1 downto 0); -- 输入时钟,可能是2MHz (2^2)
Output : out std_logic_vector(1 downto 0) -- 输出分频后的频率
);
end Counter_FrequencyDivider;
```
2. **结构体(architecture)**:
然后,定义该模块的行为。你可以通过条件分支(case statement)来实现不同频率的分频。例如,可以设置一个计数器,当 `Enable` 有效并且 `Reset` 无效时,根据 `InputClock` 的频率计算输出频率。
```vhdl
architecture Behavioral of Counter_FrequencyDivider is
type FrequencyDivisor is constant := integer range 1 to 8; -- 分频系数范围
signal DividerValue : FrequencyDivisor := 1; -- 初始分频值
begin
process(InputClock, Reset, Enable)
begin
if Reset = '1' then -- 清零
DividerValue <= 1;
elsif rising_edge(InputClock) and Enable = '1' then -- 分频操作
case InputClock(0) is
when '0' => null;
when others => DividerValue <= DividerValue * 2; -- 每次翻倍
end case;
if DividerValue = 8 then -- 达到预定输出频率时,输出并清零
Output <= (Others => '0') & not InputClock(0);
Reset <= '1';
after 1 ns Reset <= '0'; -- 等待1ns避免竞态条件
DividerValue <= 1;
else
Output <= (Others => '0') & InputClock(0); -- 其他频率时,直接输出
end if;
end if;
end process;
end Behavioral;
```
3. **LED 显示**:
分频器的输出信号 `Output` 可以连接到 LED 电路,通过点亮不同组合的LED来表示不同频率。具体连接取决于实际硬件布局。
阅读全文
相关推荐













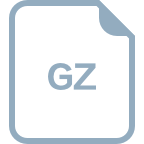




