登陆系统输入账号密码根据账号密码做比较进入系统 账号密码报错python
时间: 2024-09-14 22:14:39 浏览: 39
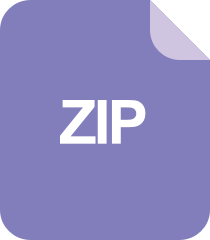
基于python用户画像的电影推荐系统的设计与实现
在Python中,登陆系统通常会涉及用户输入账户名和密码,然后与数据库存储的用户信息进行比对。这可以使用内置的数据结构如字典来模拟简单的数据存储,或连接到实际的数据库(例如MySQL、SQLite等)。以下是一个简单的流程:
```python
def login(username, password):
# 假设我们有一个预定义的用户列表或数据库查询结果
users = {"admin": "123456", "user1": "password1"} # 替换为真实的数据库查询
if username in users and users[username] == password:
print("登录成功")
# 进入系统...
else:
print("用户名或密码错误")
# 用户输入
username = input("请输入用户名:")
password = input("请输入密码:")
login(username, password)
```
在这个例子中,如果输入的`username`和`password`匹配`users`字典中的条目,登录会被认为成功;否则,会显示"用户名或密码错误"。
阅读全文
相关推荐
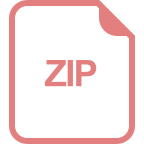
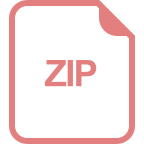

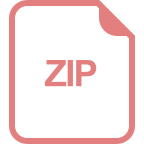
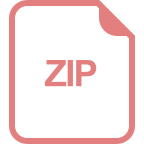
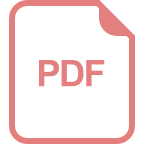
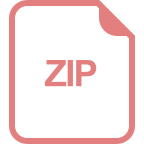
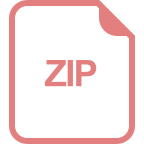
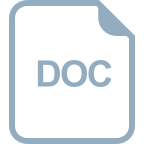
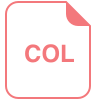
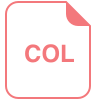
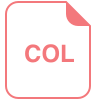





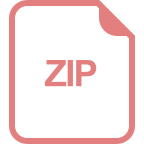
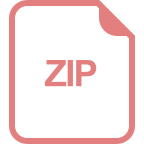