python发票管理系统
时间: 2025-01-03 21:27:07 浏览: 6
### 使用Python开发发票管理系统的教程
#### 项目概述
为了构建一个功能完善的发票管理系统,开发者可以选择基于已有的工具和技术栈来实现。该系统能够处理发票图片的导入、解析发票上的二维码信息并存储相应的数据。
#### 软件环境配置
确保本地环境中已经安装了 Python 3.x 版本以及必要的依赖库[^1]。这些依赖库包括但不限于 `os`, `logging`, `datetime`, `json`, `shutil`, `tkinter` (用于GUI界面), `windnd`(拖拽文件至窗口),还有专门用来处理 PDF 文档和条形码/QR Code 的第三方包如 PyMuPDF 和 pyzbar。
#### 创建基础结构
建立项目的根目录,在其中放置主要脚本 InvoiceMgr.py 并初始化所需的子文件夹 structure 如下:
```
/invoice_management_system/
/Output/
...
/Database/
...
config.json
InvoiceMgr.py
```
#### 编写核心逻辑
以下是简化版的核心业务流程代码片段展示如何启动程序并通过 GUI 接收用户输入的发票图像文件:
```python
import os
from tkinter import Tk, filedialog
import json
import fitz # PyMuPDF
from PIL import Image
from pyzbar.pyzbar import decode
def load_config():
with open('config.json') as f:
return json.load(f)
def save_invoice_data(data):
db_path = "Database"
if not os.path.exists(db_path):
os.makedirs(db_path)
filename = os.path.join(db_path, 'invoices.json')
try:
with open(filename, 'r+') as file:
invoices = json.loads(file.read())
invoices.append(data)
file.seek(0)
json.dump(invoices, file, indent=4)
except FileNotFoundError:
with open(filename, 'w') as file:
json.dump([data], file, indent=4)
def process_image(image_file):
image = Image.open(image_file)
decoded_objects = decode(image)
invoice_info = {}
for obj in decoded_objects:
invoice_info['type'] = str(obj.type)
invoice_info['value'] = str(obj.data.decode("utf-8"))
output_folder = "Output"
if not os.path.exists(output_folder):
os.mkdir(output_folder)
new_filename = os.path.join(output_folder, os.path.basename(image_file))
shutil.copyfile(image_file, new_filename)
save_invoice_data(invoice_info)
if __name__ == "__main__":
root = Tk()
root.withdraw() # Hide the main window.
current_directory = os.getcwd()
config = load_config()
input_dir = config.get('inputDir', '')
files = filedialog.askopenfilenames(initialdir=input_dir,
title="Select Invoice Images",
filetypes=(("jpeg files", "*.jpg"), ("all files", "*.*")))
for file in list(files):
process_image(file)
```
这段代码实现了基本的功能框架——加载配置参数、保存提取出来的发票详情到数据库中、处理上传来的每一张照片,并将其复制到指定位置以便后续查阅。
#### 扩展与优化建议
对于更复杂的需求场景,则可能涉及到更多高级特性比如 OCR 技术的应用以获取除 QR code 外的信息;或是对接官方提供的增值税发票查验服务 API 来验证票据的真实性等操作[^2][^3].
阅读全文
相关推荐









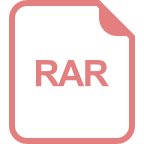








