你的不对,应该是这样的。from transformers import BertTokenizer, BertForQuestionAnswering import torch # 加载 tokenizer 和模型 tokenizer = BertTokenizer.from_pretrained('bert-base-chinese') model = BertForQuestionAnswering.from_pretrained('bert-base-chinese') # 定义问题和上下文 question = "我是谁?" context = "我是river,我在深圳,我30岁,我爱爬山。" # 对问题和上下文进行编码 inputs = tokenizer.encode_plus(question, context, add_special_tokens=True, return_tensors='pt') # 获取输入的编码表示 input_ids = inputs['input_ids'] token_type_ids = inputs['token_type_ids'] attention_mask = inputs['attention_mask'] print(input_ids) # 使用模型进行预测 output= model(input_ids=input_ids, token_type_ids=token_type_ids, attention_mask=attention_mask) start_scores = output.start_logits end_scores = output.end_logits start_index = torch.argmax(start_scores) end_index = torch.argmax(end_scores) # # 获取最高得分对应的答案位置 # start_index = torch.argmax(start_scores) # end_index = torch.argmax(end_scores) # # 使用 tokenizer 将答案位置的编码转换为文本 answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(input_ids[0][start_index:end_index+1])) print("回答:", answer)
时间: 2024-04-19 11:25:54 浏览: 30
非常抱歉之前的回答有误。您提供的代码是使用了BERT模型进行问答任务的示例,而不是Transformer模型。以下是代码的流程图:
1. 导入所需的库和模型。
2. 加载预训练的BERT模型和分词器。
3. 定义问题和上下文。
4. 使用分词器将问题和上下文编码为输入张量。
5. 从输入张量中提取编码表示。
6. 使用BERT模型进行预测,得到答案起始位置和结束位置的得分。
7. 根据得分获取最高分数对应的答案位置。
8. 使用分词器将答案位置的编码转换为文本。
9. 打印出回答的文本。
这段代码展示了如何使用BERT模型进行问答任务,使用了预训练的中文BERT模型('bert-base-chinese')进行编码和预测。
非常抱歉之前给您带来的困惑,如果还有其他问题,请随时提问。
相关问题
import torch from transformers import BertTokenizer, BertForSequenceClassification, AdamW # 加载预训练的BERT模型和tokenizer model_name = 'bert-base-uncased' tokenizer = BertTokenizer.from_pretrained(model_name) model = BertForSequenceClassification.from_pretrained(model_name, num_labels=2) # 假设是一个二分类任务 # 准备数据 df=pd.read_csv('./回复客户快捷短语.csv',encoding='gbk') train_texts=df['x'].to_list() print('打印train_list',train_texts) # 对文本进行tokenize和编码 train_encodings = tokenizer(train_texts, truncation=True, padding=True) print('打印encoding',train_encodings)
这段代码是使用PyTorch和Transformers库加载预训练的BERT模型和tokenizer,并准备数据进行编码。首先,导入必要的库,然后指定要使用的BERT模型的名称('bert-base-uncased')。接下来,使用BertTokenizer.from_pretrained()方法加载预训练的tokenizer,并使用BertForSequenceClassification.from_pretrained()方法加载预训练的BERT模型,同时指定任务的标签数量(在这里假设为二分类任务)。
然后,通过读取CSV文件获取训练文本数据train_texts。使用tokenizer对训练文本进行tokenize和编码,其中truncation参数指定是否截断文本,padding参数指定是否填充文本。
最后,打印train_texts和train_encodings的结果。train_texts是一个包含训练文本的列表,train_encodings是一个包含编码结果的字典。
from transformers import BertTokenizer, BertForQuestionAnswering import torch # 加载BERT模型和分词器 model_name = 'bert-base-uncased' tokenizer = BertTokenizer.from_pretrained(model_name) model = BertForQuestionAnswering.from_pretrained(model_name) # 输入文本和问题 context = "The Apollo program, also known as Project Apollo, was the third United States human spaceflight program carried out by the National Aeronautics and Space Administration (NASA), which succeeded in landing the first humans on the Moon from 1969 to 1972. Apollo was first conceived during the Eisenhower administration in early 1960 as a follow-up to Project Mercury. It was dedicated to President John F. Kennedy's national goal of landing Americans on the Moon before the end of the 1960s." question = "What was the goal of the Apollo program?" # 对输入进行编码 encoding = tokenizer.encode_plus(question, context, max_length=512, padding='max_length', truncation=True, return_tensors='pt') # 获取输入ids和注意力掩码 input_ids = encoding['input_ids'] attention_mask = encoding['attention_mask'] # 使用BERT模型进行问答 outputs = model(input_ids=input_ids, attention_mask=attention_mask) start_scores = outputs.start_logits end_scores = outputs.end_logits # 获取答案的起始和结束位置 start_index = torch.argmax(start_scores) end_index = torch.argmax(end_scores) # 解码答案 answer = tokenizer.convert_tokens_to_string(tokenizer.convert_ids_to_tokens(input_ids[0][start_index:end_index+1])) print(answer)
运行这段代码时,你加载了预训练的 BERT 模型和分词器,并对文本和问题进行了编码。然后,你使用 BERT 模型进行问答,通过输入输入ids和注意力掩码,模型输出了起始和结束位置的得分。接着,通过找到得分最高的位置,你解码出了答案,并将其打印出来。
请注意,这段代码还需要安装 Transformers 库和 PyTorch 库,并确保已经下载了预训练的 BERT 模型。
相关推荐












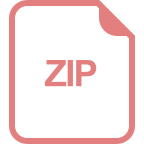