用C语言写一段代码:定义商品结构体:名称,单价,个数 1,定义函数在堆区申请空间 2,定义函数实现输入 3,计算商品总价格 4,计算最贵的商品信息 5,输入一个商品名称,查找单价信息 6,实现商品按单价排序 7,释放空间
时间: 2023-06-03 14:04:08 浏览: 62
以下是代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 商品结构体
typedef struct {
char name[50];
float price;
int quantity;
} Product;
void allocateMemory(Product **p, int n) {
// 在堆区申请空间
*p = (Product*) malloc(n * sizeof(Product));
}
void inputProducts(Product *p, int n) {
// 输入商品信息
for (int i = 0; i < n; i++) {
printf("商品 %d 的信息:\n", i + 1);
printf("名称:");
scanf("%s", (p+i)->name);
printf("单价:");
scanf("%f", &(p+i)->price);
printf("个数:");
scanf("%d", &(p+i)->quantity);
}
}
void calculateTotalPrice(Product *p, int n) {
// 计算商品总价格
float total = 0;
for (int i = 0; i < n; i++) {
total += (p+i)->price * (p+i)->quantity;
}
printf("商品总价格:%f\n", total);
}
void calculateMostExpensiveProduct(Product *p, int n) {
// 计算最贵的商品信息
float maxPrice = 0;
int index = -1;
for (int i = 0; i < n; i++) {
if ((p+i)->price > maxPrice) {
maxPrice = (p+i)->price;
index = i;
}
}
printf("最贵的商品信息:\n");
printf("名称:%s\n", (p+index)->name);
printf("单价:%f\n", (p+index)->price);
printf("个数:%d\n", (p+index)->quantity);
}
void searchProduct(Product *p, int n) {
// 输入一个商品名称,查找单价信息
char name[50];
int found = 0;
printf("请输入商品名称:");
scanf("%s", name);
for (int i = 0; i < n; i++) {
if (strcmp(name, (p+i)->name) == 0) {
printf("%s 的单价是:%f\n", name, (p+i)->price);
found = 1;
break;
}
}
if (!found) {
printf("%s 不存在!\n", name);
}
}
void sortProducts(Product *p, int n) {
// 实现商品按单价排序
for (int i = 0; i < n-1; i++) {
for (int j = i+1; j < n; j++) {
if ((p+i)->price > (p+j)->price) {
Product temp = *(p+i);
*(p+i) = *(p+j);
*(p+j) = temp;
}
}
}
printf("排序后的商品列表:\n");
for (int i = 0; i < n; i++) {
printf("商品 %d:\n", i + 1);
printf("名称:%s\n", (p+i)->name);
printf("单价:%f\n", (p+i)->price);
printf("个数:%d\n", (p+i)->quantity);
}
}
void freeMemory(Product *p) {
// 释放空间
free(p);
}
int main() {
int n;
printf("请输入商品数量:");
scanf("%d", &n);
Product *products;
allocateMemory(&products, n);
inputProducts(products, n);
calculateTotalPrice(products, n);
calculateMostExpensiveProduct(products, n);
searchProduct(products, n);
sortProducts(products, n);
freeMemory(products);
return 0;
}
相关推荐
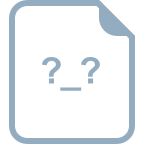
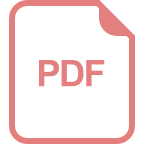
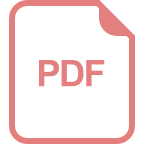
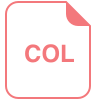
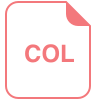
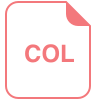
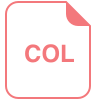
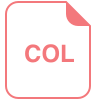









